You can convert video into black and white using OpenCV (CV2) in Python by following the given code. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision.
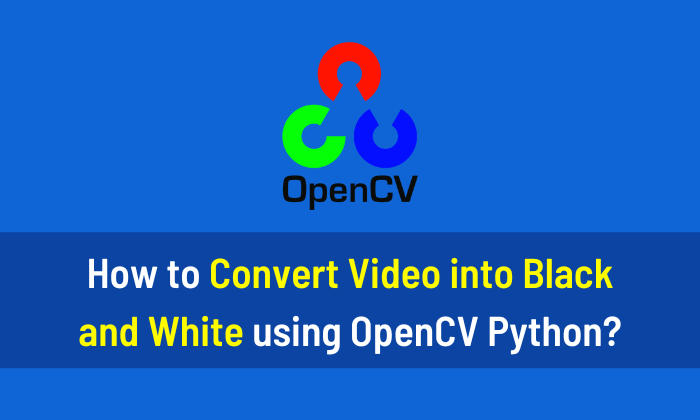
Convert Video into Black and White using CV2
If OpenCV is not installed in your system, then install it using This Method. After installation, import the required libraries. Then read the video from the location. In my case “C:\\AiHints” is the location and “cars.mp4” is the name of the video. Change it according to your video location and name. It will return the frames. Convert the frames into Grayscale then apply a threshold on it and display these frames. Press the key ‘q’ to exit the video. The function cv2.destroyAllWindows() will destroy all the windows that we created.
# Import the required Libraries import cv2 import numpy as np # Capture the video from location video = cv2.VideoCapture("C:\\AiHints\\cars.mp4") # It will return frames while (True): (ret, frame) = video.read() if not ret: print("Video Completed") break # Convert the frames into Grayscale and apply threshold gray_video = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) (thresh, binary) = cv2.threshold(gray_video, 127, 255, cv2.THRESH_BINARY) # Show the binary frames if ret == True: cv2.imshow("Car Video",binary) # Press q to exit the video if cv2.waitKey(25) & 0xFF == ord('q'): break else: break video.release() cv2.destroyAllWindows()