You can draw a bounding box in OpenCV Python by following the given steps. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision.
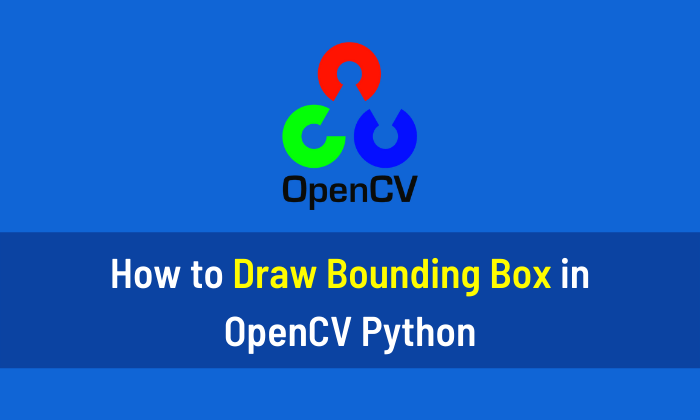
Step 1
Import the OpenCV library. If OpenCV is not installed in your system then first install it using This Method.
import cv2 #cv2 is used for OpenCV library
Step 2
Now read the image from the location. In my case “C:\\AiHints” is the location and “white.png” is the name of the image. Change it according to your image location and name.
image = cv2.imread("C:\\AiHints\\white.png") #imread is use to read an image from a location
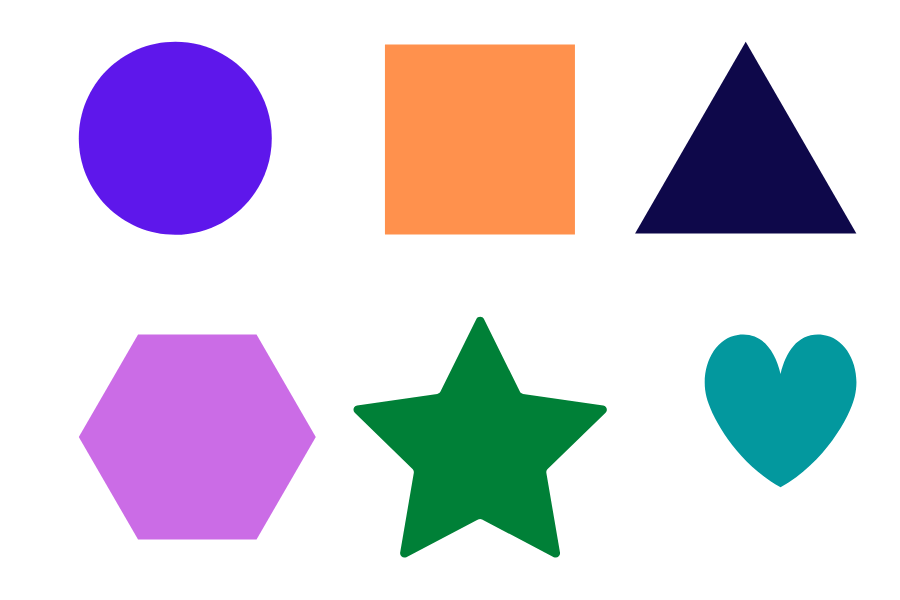
Step 3
First, convert the image into grayscale and then apply the threshold.
gray_image = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) thresh_image = cv2.threshold(gray_image, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)[1]
Step 4
Now find the contours for the objects/shapes present in the image.
contours = cv2.findContours(thresh_image, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) contours = contours[0] if len(contours) == 2 else contours[1]
Step 5
Now use the contours to draw the bounding box.
for i in contours: x,y,w,h = cv2.boundingRect(i) cv2.rectangle(img, (x, y), (x + w, y + h), (255,0,0), 4)
Step 6
To display the image in a specified window use ”imshow” function.
cv2.imshow('Bounding Box', img)
Step 7
“waitKey(0)” will display a window until any key is pressed. “destroyAllWindows()” will destroy all the windows that we created.
cv2.waitKey(0) cv2.destroyAllWindows()
Output
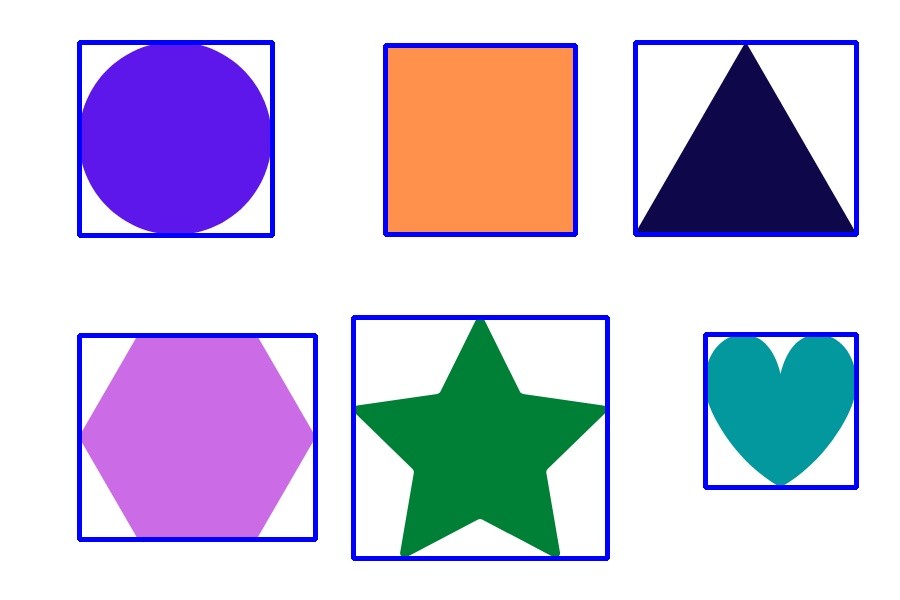
My Recommendations: Master Deep Learning with These Specializations