You can convert an image to a NumPy array in Python by following any of the given methods. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision.
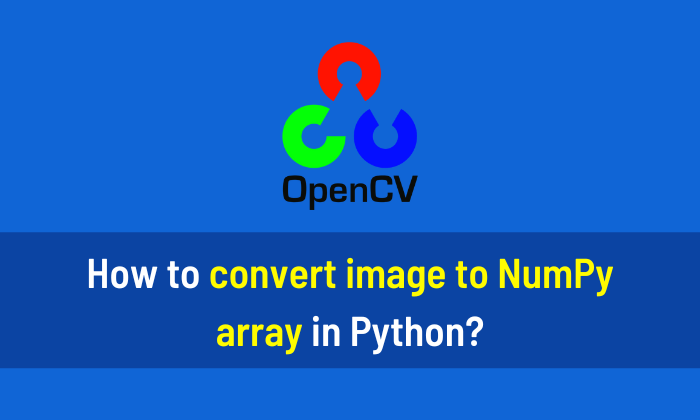
Method 1
In this method, I will use the OpenCV library to convert an image into a NumPy array. If OpenCV is not installed in your system, then install it using This Method.
import cv2 img = cv2.imread("C:\\AiHints\\cat.png") rgb_image = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) print(rgb_image)
Method 2
In this method, I will use the Pillow library to read the image. After that with the help of the NumPy library, I will convert the image into an array.
Step 1
pip install Pillow
Step 2
from PIL import Image import numpy as np image = Image.open('C:\\AiHints\\cat.png') numpy_array = np.asarray(image) print(numpy_array)
Method 3
Similar to method 2, but using np.array().
Step 1
pip install Pillow
Step 2
from PIL import Image import numpy as np image= Image.open("C:\\AiHints\\cat.png") numpy_array = np.array(image) print(numpy_array)