You can find the size of an image in Python using OpenCV by following the given steps. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision.
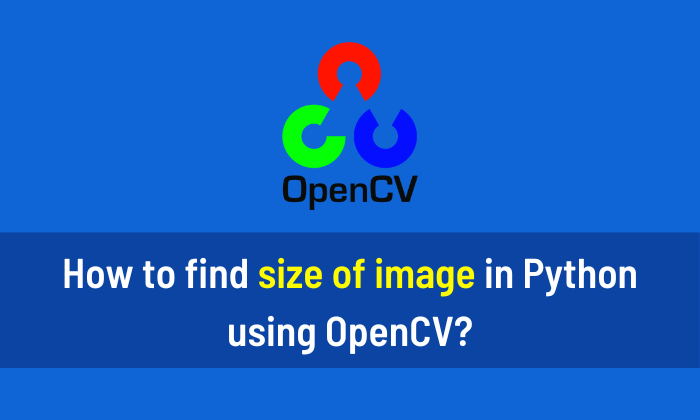
Step 1
If OpenCV is not installed, then first install it using this code.
!pip install opencv-python
Step 2
In this example, the man.jpg is the name of the image. Change it according to your image name.
# Import the OpenCV library import cv2 # Read the Image image = cv2.imread('man.jpg') # Display the size of the image print(image.size)
Output: Total Number of Pixels in the image.
4250880
Shape of Image
If you want to find the shape of the image then use this code.
import cv2 image = cv2.imread('boy.jpg') print(image.shape)
Output: Height, Width, Number of Channels
(1920, 1280, 3)