You can easily find contours in OpenCV Python with the following steps.
- Install the OpenCV library
- Import the OpenCV
- Read the Image
- Convert Image into GrayScale
- Convert Grayscale Image into Black and White
- Find Contours using function
- Draw Contours
- Display Output Image with Contours
Step 1
First, install the OpenCV library if it is not installed using this code.
pip install opencv-python
Find Contours using OpenCV
# Import the required libraries import cv2 import numpy as np # Read the image img =cv2.imread('shapes.jpg') # Convert the image into GrayScale gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # Convert the Grayscale image into Black and White _,binary_img = cv2.threshold(gray_img, 100, 255, cv2.THRESH_BINARY) # Find Contours contours, hierarchy = cv2.findContours(binary_img, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) # Draw Contours drawing = np.zeros((gray_img.shape[0], gray_img.shape[1], 3), dtype=np.uint8) countours_img = cv2.drawContours(drawing,contours, -1, (255,255,0),3) # Display the Output cv2.imshow('Original Image',img) cv2.imshow('Image with Contours',countours_img ) # Destroy all the windows cv2.waitKey(0) cv2.destroyAllWindows()
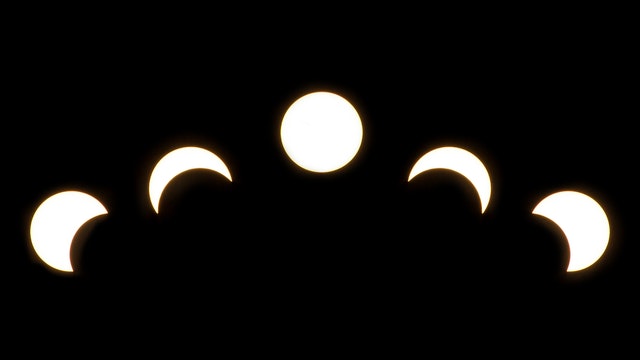
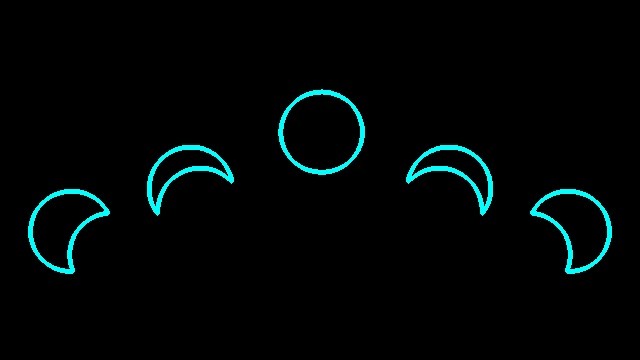
I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision.