You can get indices of n maximum values in a NumPy array with the following code. If you want to learn Python, I highly recommend reading This Book.
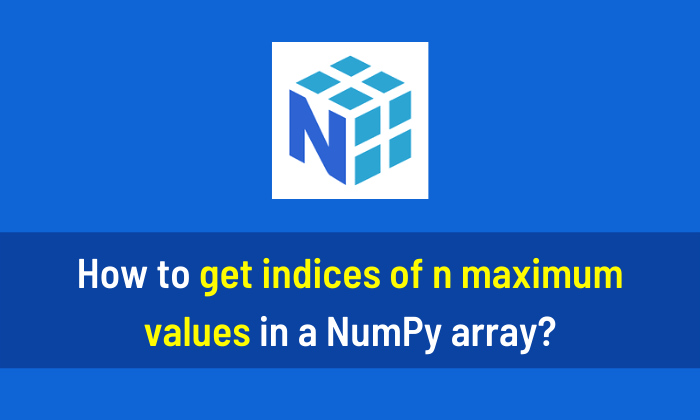
Example
In this example, I will get indices of 4 maximum values of a NumPy array.
# Import numpy library import numpy as np # Initialize a numpy array a = np.array([8, 2, 5, 7, 6, 1]) # Get indices of 4 maximum values from the array b = np.argpartition(a, -4)[-4:] # Sort the indices according to maximum values indices = b[np.argsort((-a)[b])] # Display the indices print(indices)
Output:
[0 3 4 2]
People are also reading:
What is Computer Vision? Examples, Applications, Techniques
Books for Machine Learning (ML)