In this article, you’ll see how to create a water effect in Python using OpenCV. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision. To create a water effect just follow these steps:
Step 1: Install OpenCV
If OpenCV is not installed, then first install it using this code.
pip install opencv-python
Step 2: Import the required Libraries
Import the OpenCV and NumPy libraries.
import cv2 import numpy as np
Step 3: Read the Image
Now read the image from the location. ‘animal.jpg‘ is the name of the image.
img = cv2.imread("C:\\AiHints\\animal.jpg")
Step 4: Flip the Image
In this step, flip the image vertically.
flip_img = cv2.flip(img, 0)
Step 5: Create a water effect in Python
Now, simply create a water effect using the concatenation of the above two images vertically.
water_effect = np.concatenate((img, flip_img), axis=0)
Step 6: Display the water effect
Now display the original, flipped, and water effect images.
cv2.imshow("Original Image", img) cv2.imshow("Flipped Image", flip_img) cv2.imshow("Water Effect", water_effect) cv2.waitKey(0) cv2.destroyAllWindows()
Output:
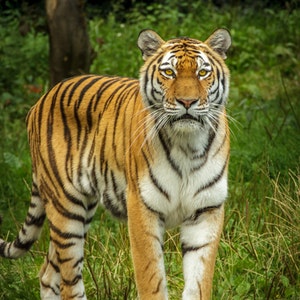
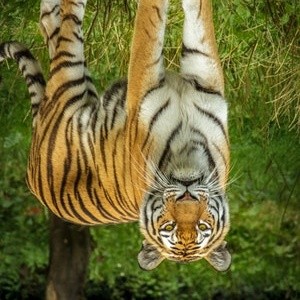
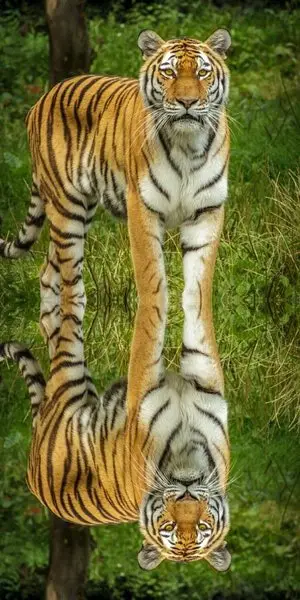