You can convert a color image in grayscale using OpenCV Python by following the given steps. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision.
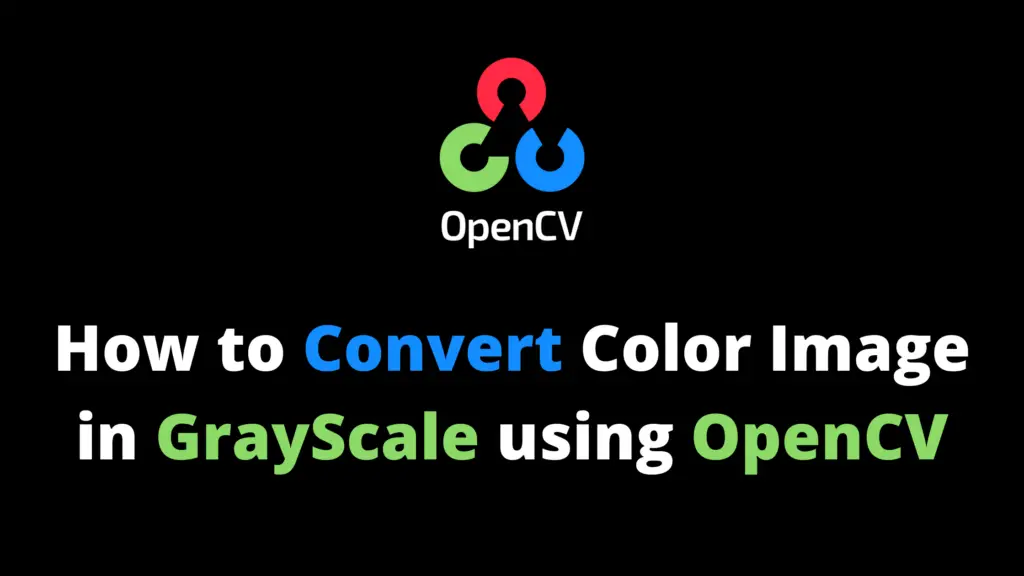
Step 1
Open the Spyder IDE (integrated development environment).
Step 2
Import the OpenCV library. If OpenCV is not installed in your system then first install it using This Method.
import cv2 #cv2 is used for OpenCV library
Step 3
Now read the image from the location. In my case “F:\\AiHints” is the location and “top30.png” is the name of the image. Change it according to your image location and name. In this code, “0” will convert the color image into GrayScale.
image = cv2.imread("F:\\AiHints\\top30.png",0) #imread is use to read an image from a location
Step 4
In this step, we will open the image in a new window with the name “Gray Scale Image” then print this image in the form of a two-dimensional array.
cv2.imshow("Gray Scale Image",image) #imshow command is use to show an image print("Image in Gray Scale==\n",image)
Step 5
waitKey() open the image for a specific time in milliseconds until you press any key. “destroyAllWindows()” will destroy all the windows that we created.
cv2.waitKey(0) cv2.destroyAllWindows()
Method 2
import cv2 img = cv2.imread("F:\\AiHints\\top30.png") gray_image = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) cv2.imshow("Gray Scale Image",gray_image) print("Image in Gray Scale==\n",gray_image) cv2.waitKey(0) cv2.destroyAllWindows()