You can translate an image using OpenCV Python by following the given steps. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision.
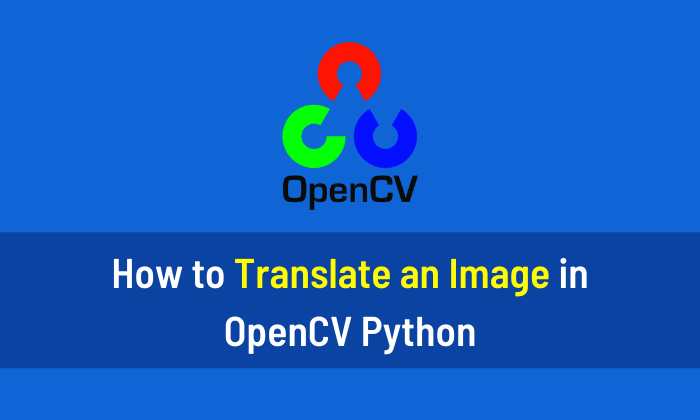
Step 1
Import the required libraries. If OpenCV is not installed in your system then first install it using This Method.
import cv2 #cv2 is used for OpenCV library import numpy as np
Step 2
Now Read the Image. The image should be in the current working directory. Otherwise, mention the location of the image.
img =cv2.imread('cat.jpg')
Step 3
Now make a matrix for translation. You can change the translation values. I will translate the image 160 units along the x-axis and 80 units along the y-axis. 1,0 and 0,1 is because there is no rotation of image.
rows, cols = img.shape[:2] matrix = np.float32([[1, 0, 160], [0, 1, 80]])
Step 4
Now apply the above matrix to the image and use the method cv2.warpAffine.
translated_img = cv2.warpAffine(img, matrix, (cols, rows))
Step 5
Now display both images.
cv2.imshow('Cat',img) cv2.imshow('Translated Image',translated_img) cv2.waitKey(0) cv2.destroyAllWindows()
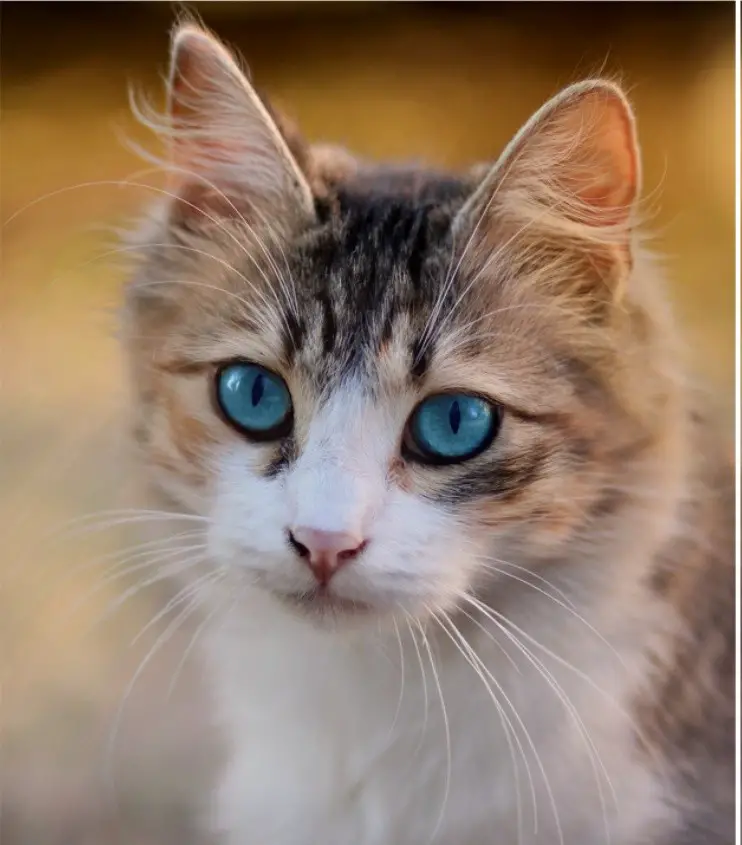
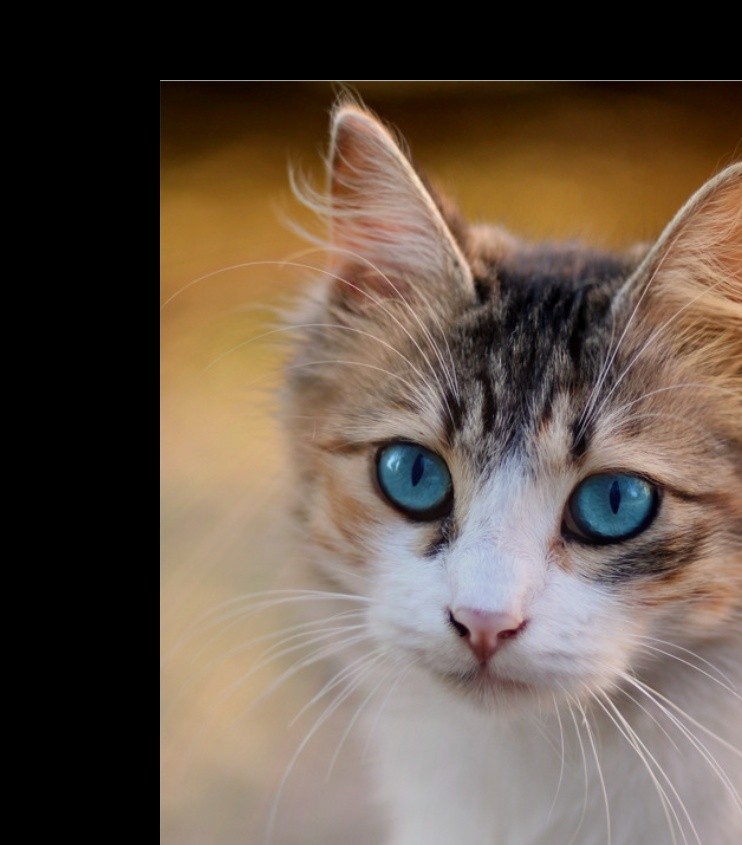