In this OpenCV Tutorial, you’ll learn how to perform template matching in OpenCV Python. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn computer vision. We will use the following two images for template matching.
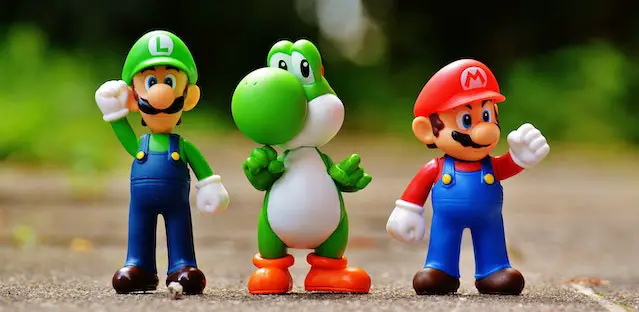
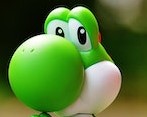
# Import the required libraries import cv2 import numpy as np # Read an image img = cv2.imread("C:\\aihints\\cartoon.jpg") # Read template image img_2 = cv2.imread("C:\\aihints\\part.jpg") # Get width and height of template image width, height = img_2.shape[:-1] # Template matching tm = cv2.matchTemplate(img, img_2, cv2.TM_CCOEFF_NORMED) # Set a Threshold threshold = 0.8 # Store the coordinates of matched area in a numpy array area = np.where(tm >= threshold) # Draw a rectangle around the matched region. for i in zip(*area[::-1]): cv2.rectangle(img, i, (i[0] + width, i[1] + height), (255, 0, 0), 1) # Display the template matching image cv2.imshow('Template Matching', img) # Save the template matching image cv2.imwrite('C:\\AiHints\\template_matching.jpg', img) # Wait until any key is pressed cv2.waitKey(0) # Close all the windows cv2.destroyAllWindows()
Output:
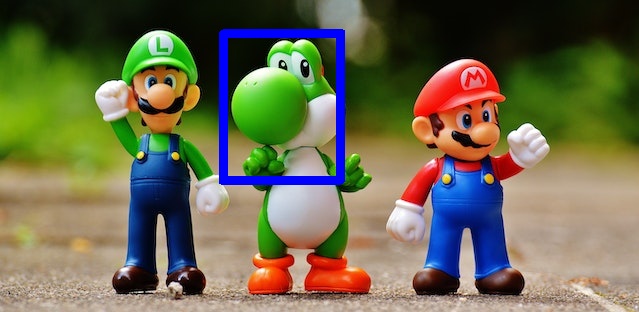