You can print the image pixels in Python by following the given steps. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision.
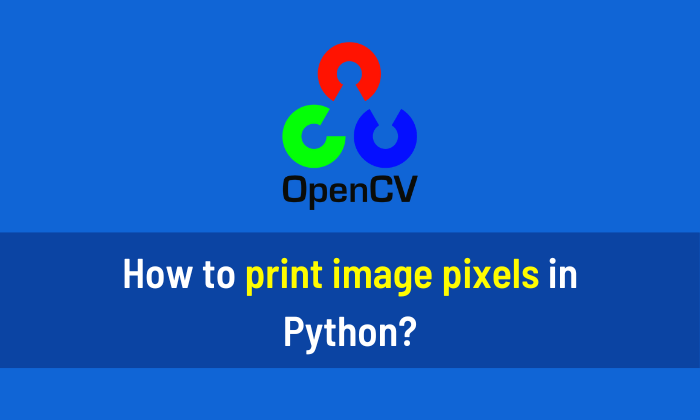
Step 1
If OpenCV is not installed, then first install it using this code.
!pip install opencv-python
Step 2
In this example, the man.jpg is the name of the image. Change it according to your image name. The image should be in the same folder in which your code file is running.
# Import the OpenCV library import cv2 # Read the Image image = cv2.imread('man.jpg') # Print Image Pixels print(image)
Output:
[[[188 174 108] [197 182 119] [205 192 130] ... [200 185 113] [192 177 104] [169 154 81]] [[191 176 107] [199 183 116] [209 194 131] ... [172 156 90] [158 143 80] [138 123 60]] [[198 184 108] [201 187 111] [211 196 124] ... [120 106 54] [114 100 52] [104 91 45]] ... [[179 169 145] [177 168 141] [174 166 137] ... [ 96 78 41] [ 81 71 61] [ 79 72 75]] [[178 169 142] [177 169 140] [173 165 136] ... [ 88 70 47] [ 70 59 61] [ 66 59 74]] [[178 169 142] [177 169 140] [173 165 136] ... [ 82 63 50] [ 60 48 60] [ 52 44 67]]]
Print Specific Single Pixel
In this example, I will print the specific pixel of the image.
# Import the OpenCV library import cv2 # Read the Image image = cv2.imread('man.jpg') # Print Image Specific Pixel print(image[0][0])
Output:
[188 174 108]