You can do perspective transformation using OpenCV Python by following the given steps. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision.
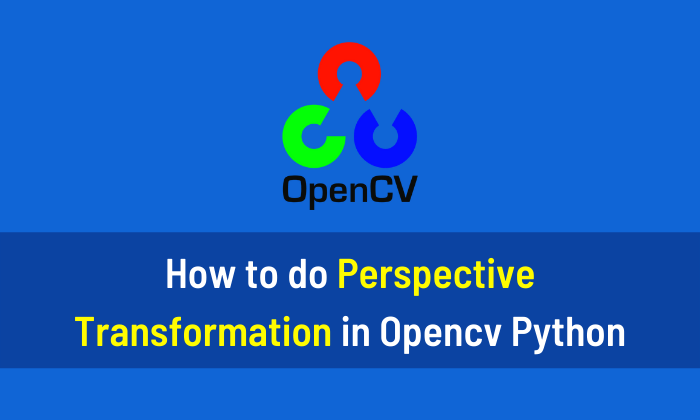
Step 1
Import the required libraries. If OpenCV is not installed in your system then first install it using This Method.
import cv2 #cv2 is used for OpenCV library import numpy as np
Step 2
Now Read the Image. The image should be in the current working directory. Otherwise, mention the location of the image.
img =cv2.imread('cat.jpg')
Step 3
Now make two variables rows and cols. Use these two variables to make the final points of the required image.
rows, cols = img.shape[:2] initial_points = np.float32([[0,0],[cols-1,0],[0,rows-1],[cols-1,rows-1]]) final_points = np.float32([[0,0],[cols-1,0],[int(0.30*cols),rows-1],[int(0.60*cols),rows-1]])
Step 4
First, make a matrix using the function cv2.getPerspectiveTransform. Then apply the matrix to the image using the function cv2.warpPerspective.
matrix = cv2.getPerspectiveTransform(initial_points,final_points) perspective = cv2.warpPerspective(img,matrix,(cols,rows))
Step 5
Now display both images.
cv2.imshow('Cat',img) cv2.imshow('Perspective Transformation',perspective) cv2.waitKey(0) cv2.destroyAllWindows()
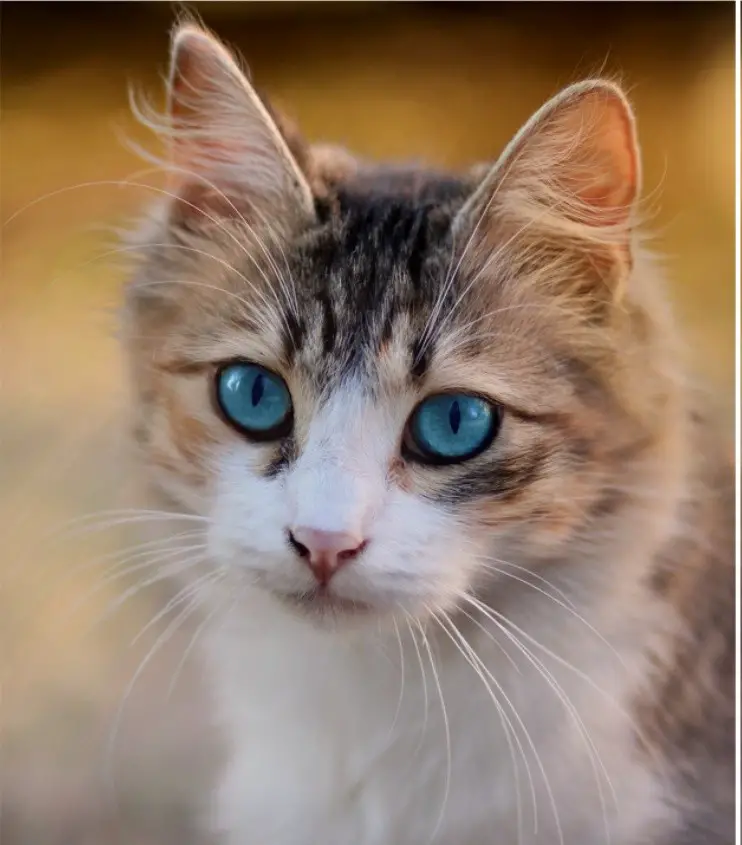
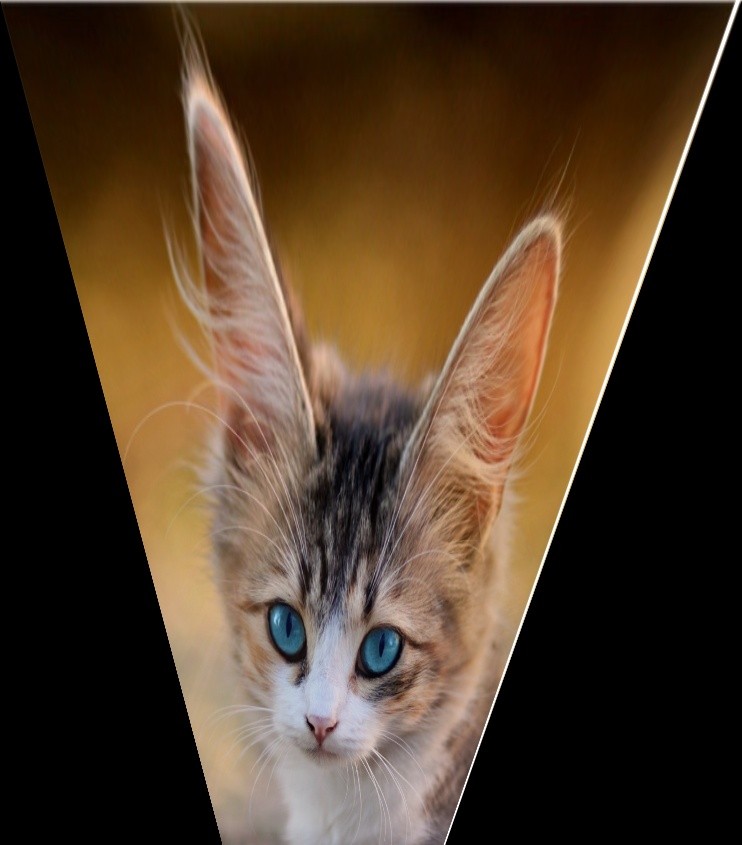