You can do Euclidean Transformation using OpenCV Python by following the given steps. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision.
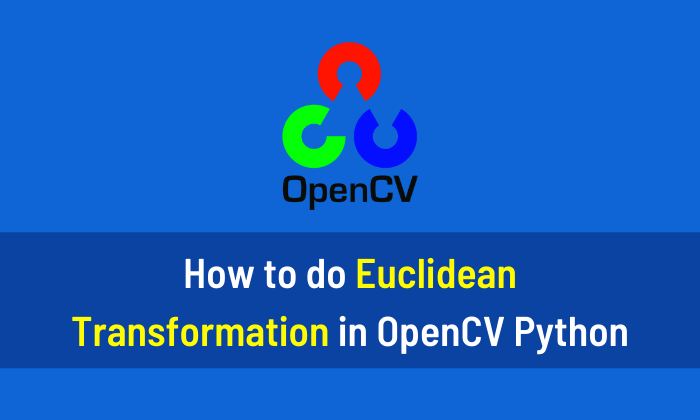
Step 1
Import the required libraries. If OpenCV is not installed in your system then first install it using This Method.
import cv2 #cv2 is used for OpenCV library import numpy as np
Step 2
Now Read the Image. The image should be in the current working directory. Otherwise, mention the location of the image.
img =cv2.imread('cat.png')
Step 3
Now make a matrix for rotation and translation. You can change the values according to rotation angle and translation. The value of Cos30 degree is 0.86 and the value of Sin30 degree is 0.5. The translation value along the x-axis is 200 and along the y-axis is 20.
matrix = np.float32([[0.86,-0.5,200],[0.5,0.86,20]])
Step 4
Now apply the above matrix to the image and use the method cv2.warpAffine.
euclidean = cv2.warpAffine(img,matrix,(img.shape[1]+100,img.shape[0]+100))
Step 5
Now display both images.
cv2.imshow('Cat',img) cv2.imshow('Euclidean Transformation',euclidean) cv2.waitKey(0) cv2.destroyAllWindows()
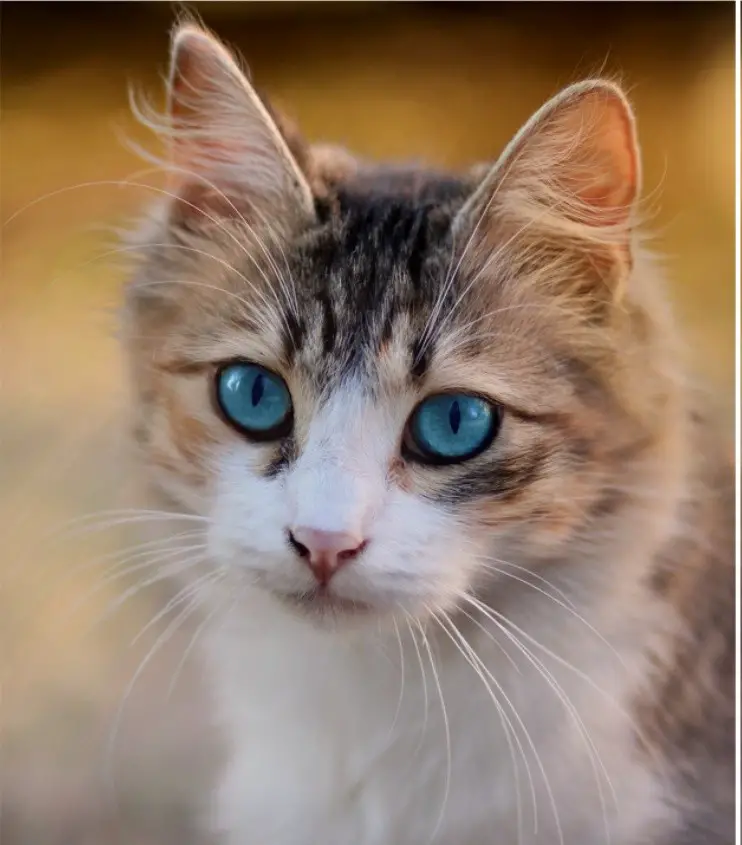
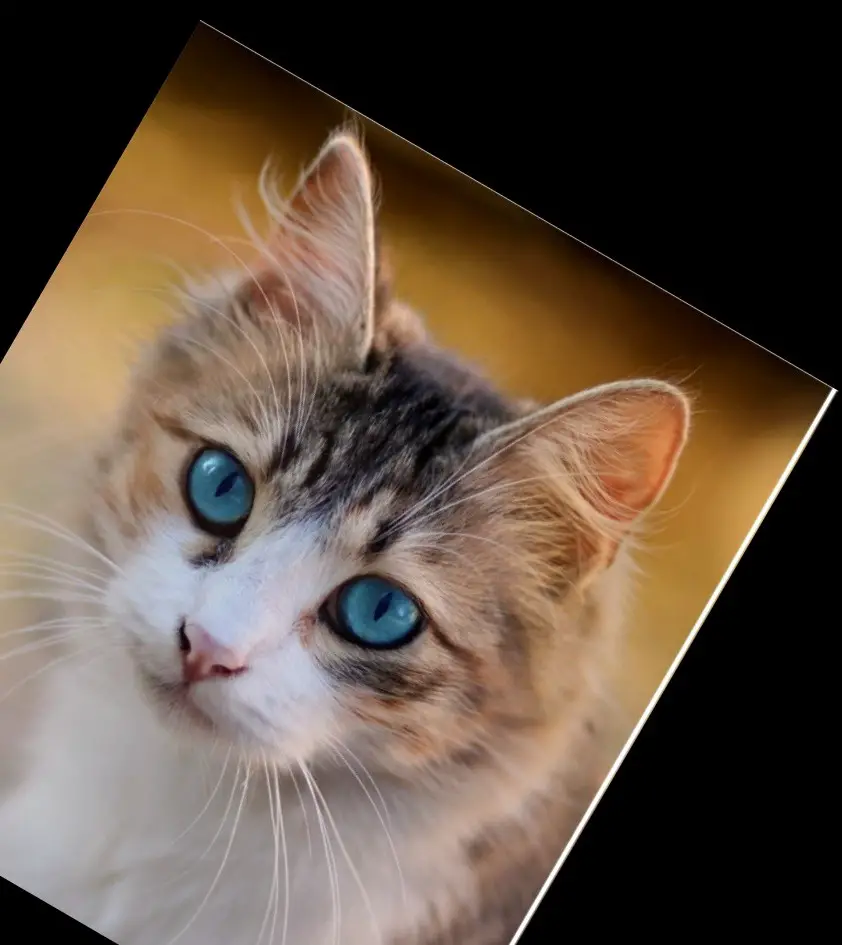