You can convert color video into grayscale using OpenCV (CV2) in Python by following the given code. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision.
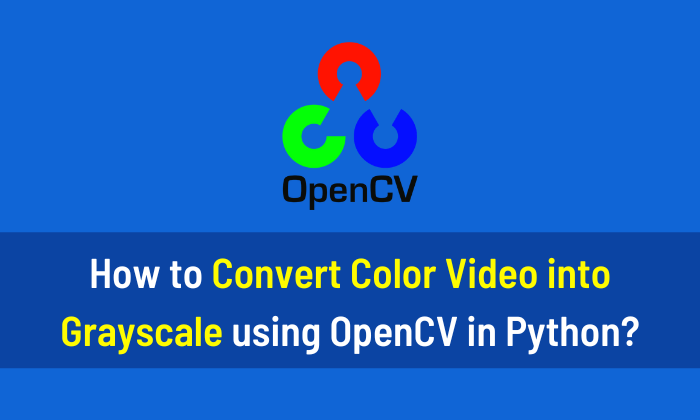
Convert Color Video into Grayscale using CV2
If OpenCV is not installed in your system, then install it using This Method. After installation, import the required libraries. Then read the video from the location. In my case “C:\\AiHints” is the location and “cars.mp4” is the name of the video. Change it according to your video location and name. It will return the frames. Convert the frames into Grayscale and display these grayscale frames. Press the key ‘q’ to exit the video. The function cv2.destroyAllWindows() will destroy all the windows that we created.
# Import the required Libraries import numpy as np import cv2 # Capture the video from location video = cv2.VideoCapture("C:\\AiHints\\cars.mp4") # It will return frames while video.isOpened(): ret, frame = video.read() if not ret: print("Video Completed") break # Convert the frames into Grayscale gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) # Show the Grayscale frames cv2.imshow("Car Video",gray) # Press q to exit the video if cv2.waitKey(25) & 0xFF == ord('q'): break video.release() cv2.destroyAllWindows()