In this article, you’ll see how to split an image into its 3 RGB channels using OpenCV in Python. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision. Just follow these steps:
Step 1: Install OpenCV
If OpenCV is not installed, then first install it using this code.
pip install opencv-python
Step 2: Import OpenCV
Import the OpenCV library.
import cv2
Step 3: Read the Image
Now read the image from the location.
image = cv2.imread("C:\\AiHints\\opencv.png")
Step 4: Split Image into Channels
In this step, we will split the image using cv2.split() function.
blue, green, red = cv2.split(image)
Step 5: Display the split channels
Now display the result.
cv2.imshow("Original Image", image) cv2.imshow("Blue Image", blue) cv2.imshow("Green Image", green) cv2.imshow("Red Image", red) cv2.waitKey(0) cv2.destroyAllWindows()
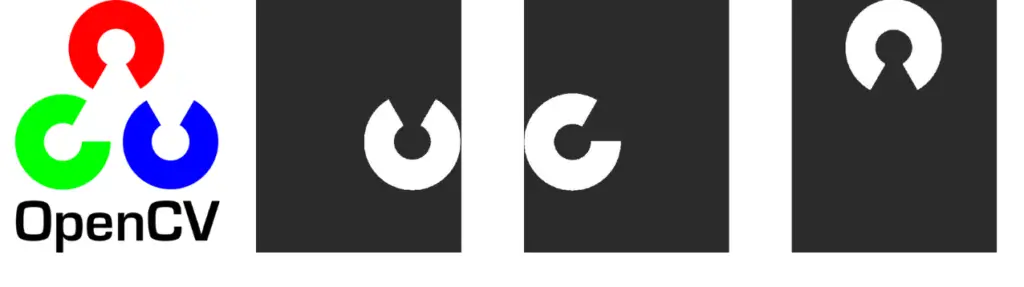
Complete Code
import cv2 image = cv2.imread("C:\\AiHints\\opencv.png") blue, green, red = cv2.split(image) cv2.imshow("Original Image", image) cv2.imshow("Blue Image", blue) cv2.imshow("Green Image", green) cv2.imshow("Red Image", red) cv2.waitKey(0) cv2.destroyAllWindows()