In this OpenCV Tutorial, you’ll learn how to perform SIFT in OpenCV Python. SIFT is a short form of Scale Invariant Fourier Transform. This is a detector that is used for the detection of interest points in an image. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn computer vision. I will use the following image.
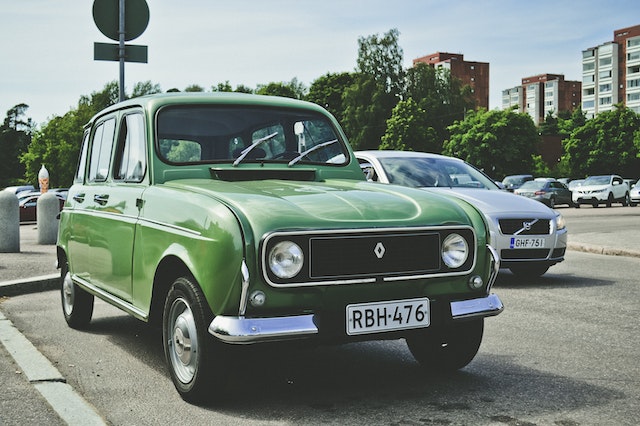
# import OpenCV module import cv2 # Read the image img = cv2.imread('C:\\AiHints\\car.jpg') # Convert the image to grayscale gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # Create a SIFT object sift = cv2.SIFT_create() # Detect keypoints keypoints = sift.detect(gray_img, None) # Draw the keypoints on the image # You can remove the last parameter that is used for direction img = cv2.drawKeypoints(gray_img, keypoints, img, flags=cv2.DRAW_MATCHES_FLAGS_DRAW_RICH_KEYPOINTS) # Display the image cv2.imshow('Final Image', img) # Save the image cv2.imwrite('C:\\AiHints\\car_sift.jpg', img) # Wait until a key is pressed cv2.waitKey(0) # Destroy all windows cv2.destroyAllWindows()
Output:
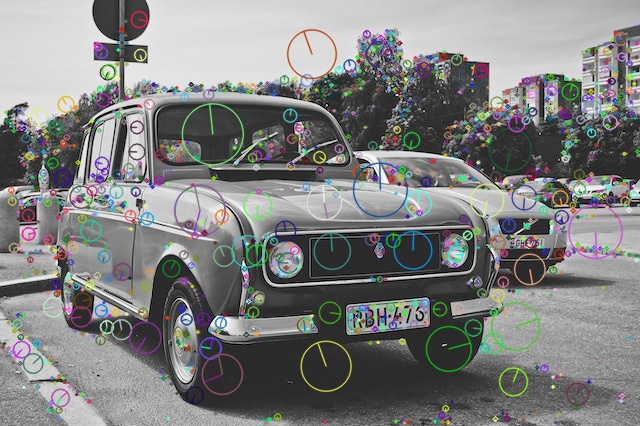