In this article, you’ll see how to create a mirror image in Python using OpenCV. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision. To create a mirror image in Python just follow these steps:
Step 1: Install OpenCV
If OpenCV is not installed, then first install it using this code.
pip install opencv-python
Step 2: Import the required Libraries
Import the OpenCV and NumPy libraries.
import cv2 import numpy as np
Step 3: Read the Image
Now read the image from the location. ‘man.jpg‘ is the name of the image.
image = cv2.imread("C:\\AiHints\\man.jpg")
Step 4: Flip the Image Horizontally
In this step, flip the original image horizontally.
h_flip = cv2.flip(image, 1)
Step 5: Create a Mirror Image
Now, simply create a mirror effect using the concatenation of the above two images horizontally.
mirror_image = np.concatenate((image, h_flip), axis=1)
Step 6: Display the Mirror Image
Now display the original, flipped, and mirror images.
cv2.imshow("Original Image", image) cv2.imshow("Flipped Image", h_flip) cv2.imshow("Mirror Image", mirror_image) cv2.waitKey(0) cv2.destroyAllWindows()
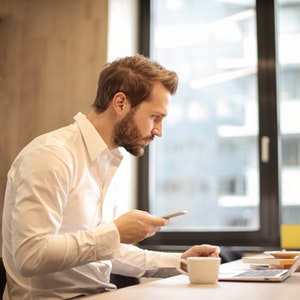
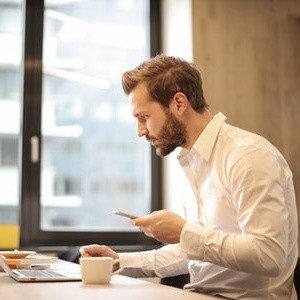
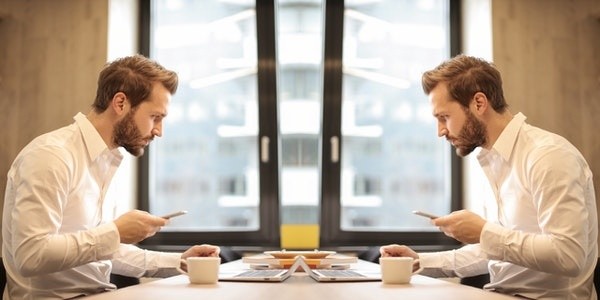