In this article, you’ll see image subtraction in Python using OpenCV. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision. For image subtraction in Python just follow these steps:
Step 1: Install OpenCV
If OpenCV is not installed, then first install it using this code.
pip install opencv-python
Step 2: Import OpenCV
Import the OpenCV library.
import cv2
Step 3: Read the Images
Now read the images from the location.
img1 = cv2.imread("C:\\AiHints\\image1.png") img2 = cv2.imread("C:\\AiHints\\image2.png")
Step 4: Image Subtraction
In this step, subtract the images using cv2.subtract() function.
sub_img = cv2.subtract(img1,img2)
Step 5: Display the Output
cv2.imshow("Image 1", img1) cv2.imshow("Image 2", img2) cv2.imshow("Image Subtraction", sub_img) cv2.waitKey(0) cv2.destroyAllWindows()
Output:
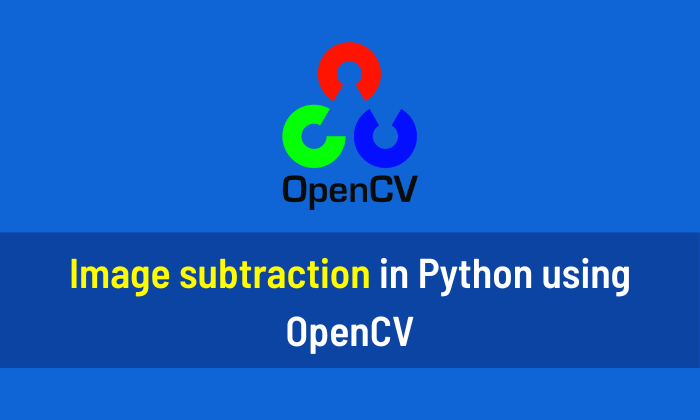
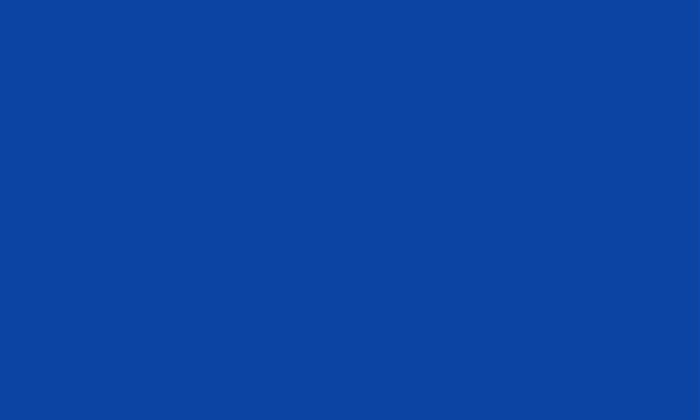
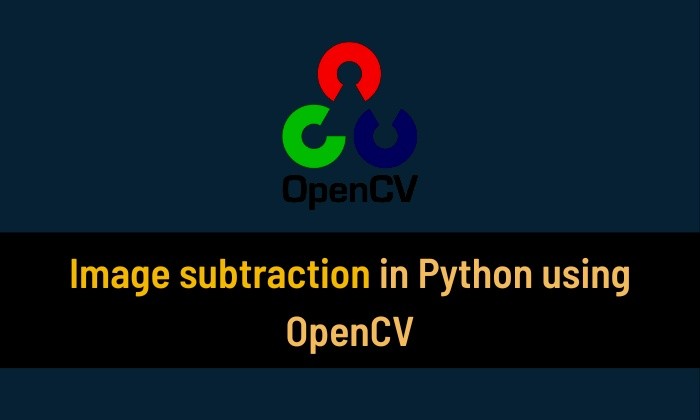