You can find the total number of contours in OpenCV Python with the following steps.
- Install the OpenCV
- Import the OpenCV
- Read the Image
- Convert the Image into GrayScale
- Convert the Grayscale Image into a Black and White Image
- Find the Contours using the function
- Draw the Contours (Optional)
- Find Number of Contours
- Display Output Image with Contours (Optional)
Step 1
First, install the OpenCV library using this code if it is not installed.
pip install opencv-python
Find the number of Contours in OpenCV
# Import the required libraries import cv2 import numpy as np # Read the image image =cv2.imread('shapes.jpg') # Convert the image into GrayScale gray_img = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) # Convert the Grayscale image into Black and White _,binary_img = cv2.threshold(gray_img, 100, 255, cv2.THRESH_BINARY) # Find Contours contours, hierarchy = cv2.findContours(binary_img, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) # Draw Contours drawing = np.zeros((gray_img.shape[0], gray_img.shape[1], 3), dtype=np.uint8) countours_img = cv2.drawContours(drawing,contours, -1, (255,255,0),3) # Number of Contours total_contours = len(contours) print(total_contours) # Display the Original Image and Output cv2.imshow('Original Image',image) cv2.imshow('Image with Contours',countours_img) # Destroy all the windows cv2.waitKey(0) cv2.destroyAllWindows()
Output:
5
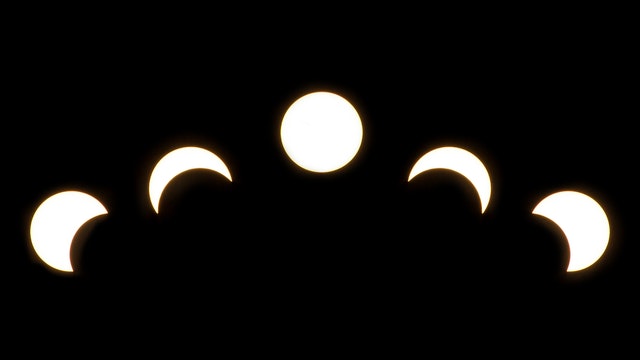
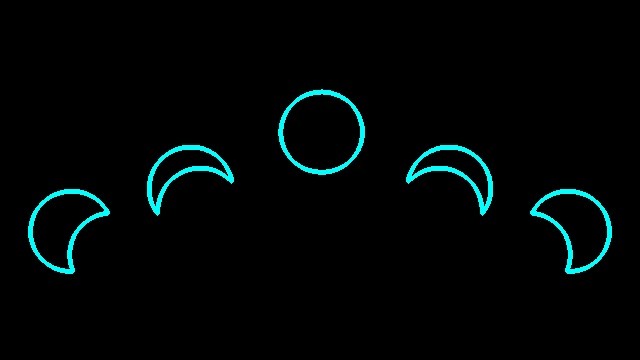
I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision.