You can draw a filled circle in OpenCV Python by following the given steps. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision.
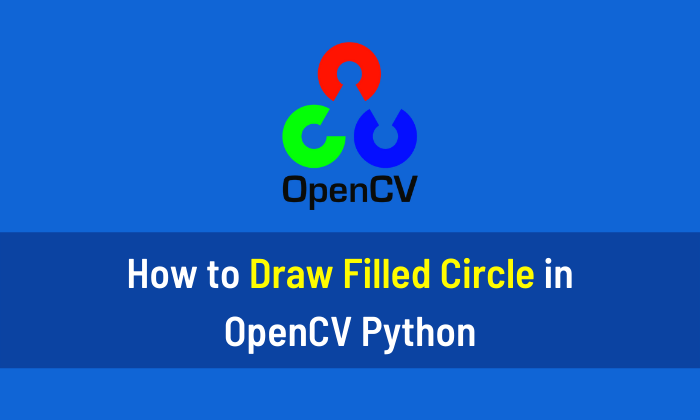
Step 1
Import the OpenCV library. If OpenCV is not installed in your system then first install it using This Method.
import cv2 #cv2 is used for OpenCV library
Step 2
Now read the image from the location. In my case “C:\\AiHints” is the location and “AI.jpg” is the name of the image. Change it according to your image location and name.
image = cv2.imread("C:\\AiHints\\AI.jpg") #imread is use to read an image from a location
Step 3
Now I will draw a filled circle on the image using the following code. In the code, (80,80) is the center point, and 50 is the diameter of the circle. After that, I will specify a color for the circle (0,255,0) that is green color. The last parameter in both methods is for the filled circle. You can change any value according to your requirement.
# Both methods will give same results. You can use any method to draw filled circle. image2 = cv2.circle(image,(80,80), 50, (0,255,0),-1) #Method 1 image2 = cv2.circle(image,(80,80), 50, (0,255,0),cv2.FILLED) #Method 2
Step 4
To display the image in a specified window use ”imshow” function.
cv2.imshow("Green Filled Circle on Image",image2)
Step 5
“waitKey(0)” will display a window until any key is pressed. “destroyAllWindows()” will destroy all the windows that we created.
cv2.waitKey(0) cv2.destroyAllWindows()
Output
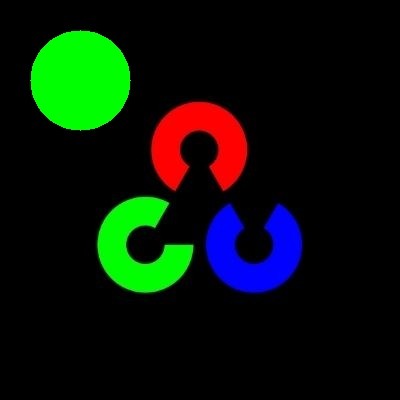