In this article, you’ll see how to convert a grayscale image to a binary image in OpenCV. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision. For converting a grayscale image to a binary image just follow these steps:
Step 1: Install OpenCV
If OpenCV is not installed, then first install it using this code.
pip install opencv-python
Step 2: Import the OpenCV library
import cv2
Step 3: Read the Grayscale Image
Now read the image from the location.
image = cv2.imread("C:\\AiHints\\gray_cat.jpg")
Step 4: Grayscale to Binary
Now, convert the grayscale image into binary.
thresh, binary = cv2.threshold(image, 150, 255, cv2.THRESH_BINARY)
Step 5: Output
Now, display the grayscale image and binary image.
cv2.imshow("Gray Image", image) cv2.imshow("Binary Image", binary) cv2.waitKey(0) cv2.destroyAllWindows()
Output:
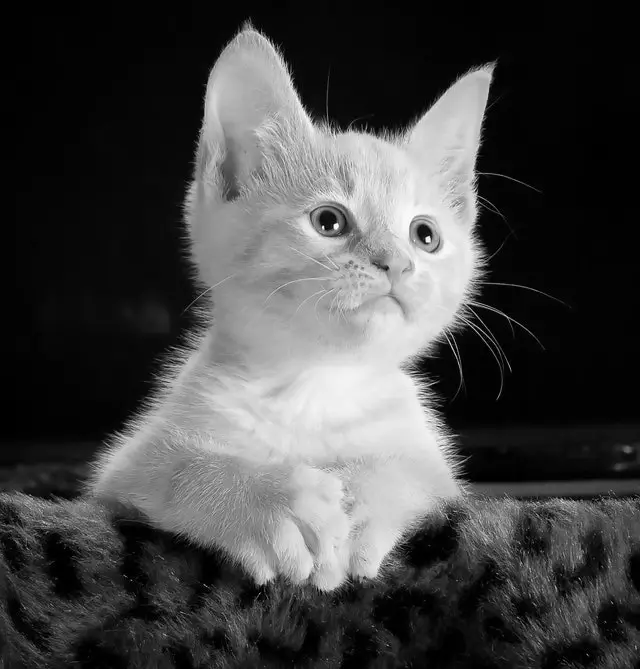
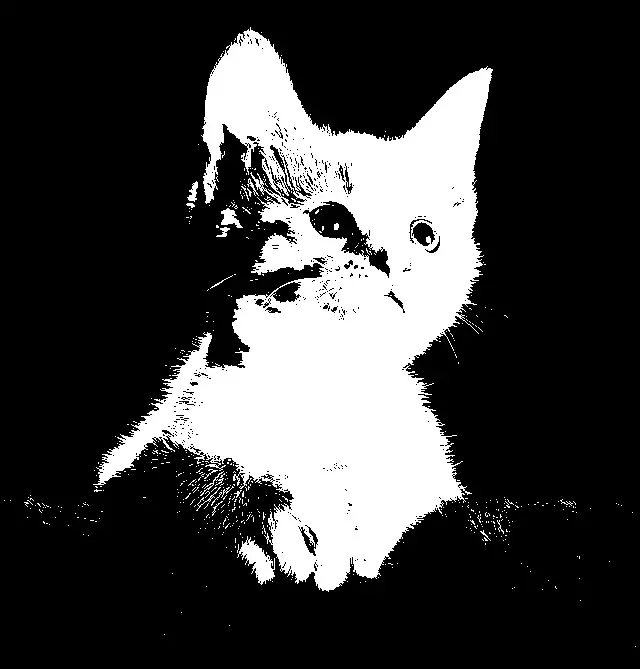