In this OpenCV Tutorial, you’ll learn how to implement edge detection in OpenCV Python. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn computer vision. These two following edge-detection algorithms are available in OpenCV. We will implement both algorithms on the following image and see their results.
- Canny Edge Detection
- Sobel Edge Detection
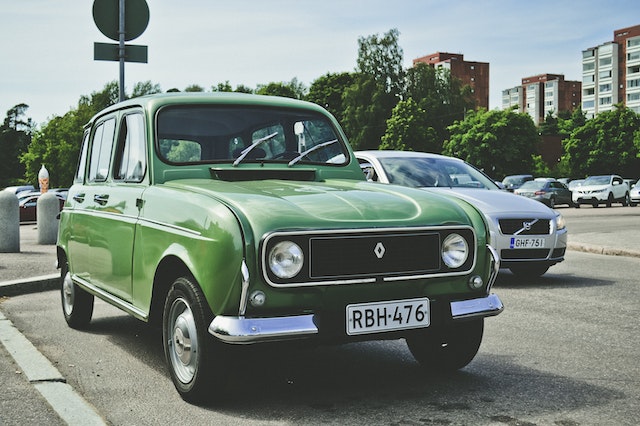
Canny Edge Detection
You need to just change the name and location of the image.
# Import the OpenCV library import cv2 # Read the original image from the location img = cv2.imread('C:\\AiHints\\car.jpg') # Convert the image into graycsale gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # Blur the gray scale image for better edge detection blur = cv2.GaussianBlur(gray, (5,5), 0) # Canny Edge Detection canny_edges = cv2.Canny(image=blur, threshold1=100, threshold2=200) # Display Canny Edge Detection Image cv2.imshow('Canny Edge Detection', canny_edges) cv2.waitKey(0) cv2.destroyAllWindows() # Save the Canny Edge Detection Image cv2.imwrite('C:\\AiHints\\canny.jpg', canny_edges)
Output:
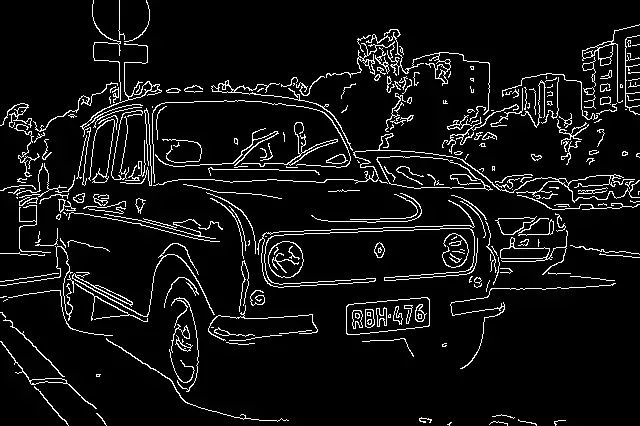
Sobel Edge Detection
# Import the OpenCV library import cv2 # Read the image from the location img = cv2.imread('C:\\AiHints\\car.jpg') # Convert the image into graycsale gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # Blur the gray scale image for better edge detection blur = cv2.GaussianBlur(gray, (5,5), 0) # Sobel Edge Detection on x-axis sobelx = cv2.Sobel(src=blur, ddepth=cv2.CV_64F, dx=1, dy=0, ksize=5) # Sobel Edge Detection on y-axis sobely = cv2.Sobel(src=blur, ddepth=cv2.CV_64F, dx=0, dy=1, ksize=5) # Sobel Edge Detection on both axis sobelxy = cv2.Sobel(src=blur, ddepth=cv2.CV_64F, dx=1, dy=1, ksize=5) # Display Sobel Edge Detection Images cv2.imshow('Sobel X Edge Detection', sobelx) cv2.imshow('Sobel Y Edge Detection', sobely) cv2.imshow('Sobel X and Y Edge Detection', sobelxy) cv2.waitKey(0) cv2.destroyAllWindows() # Save the Sobel Edge Detection Images cv2.imwrite('C:\\AiHints\\sobelx.jpg', sobelx) cv2.imwrite('C:\\AiHints\\sobely.jpg', sobely) cv2.imwrite('C:\\AiHints\\sobelxy.jpg', sobelxy)
Output:
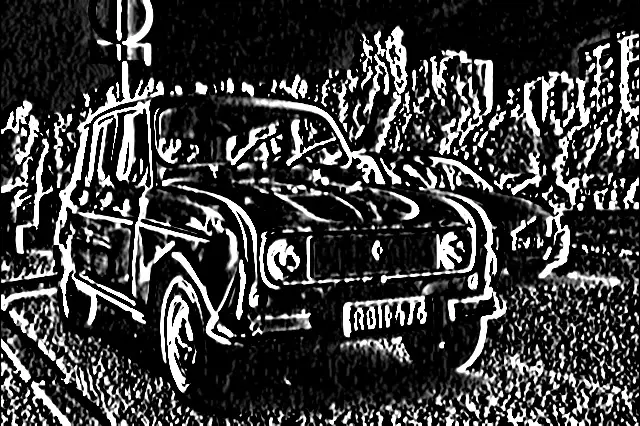
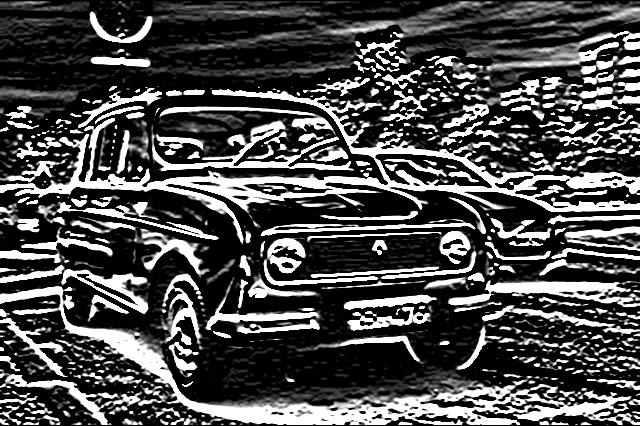
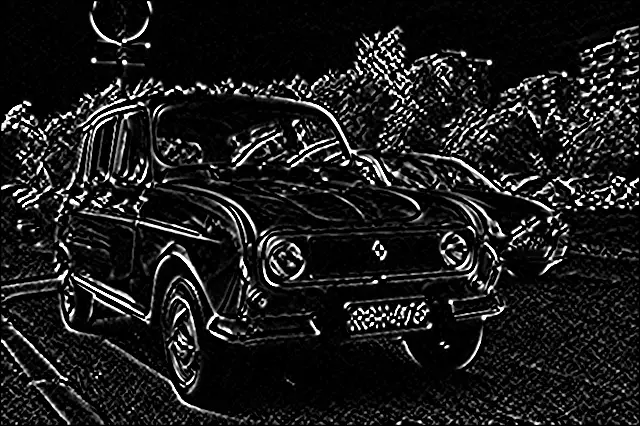