In this article, you’ll see how to convert HSV to RGB in OpenCV. I highly recommend you get the “Computer Vision: Models, Learning, and Inference Book” to learn Computer Vision. You need to just follow these steps:
Step 1: Install OpenCV
If OpenCV is not installed, then first install it using this code.
pip install opencv-python
Step 2: Import the OpenCV library
import cv2
Step 3: Read the HSV Image
Now read the HSV image from the location.
image = cv2.imread("C:\\AiHints\\hsv_image.jpg")
Step 4: Convert into RGB
In this step, convert the image from HSV to RGB using cv2.cvtColor() function.
rgb_image = cv2.cvtColor(image, cv2.COLOR_HSV2RGB)
Step 5: Display Output
cv2.imshow("HSV Image", image) cv2.imshow("RGB Image", rgb_image) cv2.waitKey(0) cv2.destroyAllWindows()
Output:
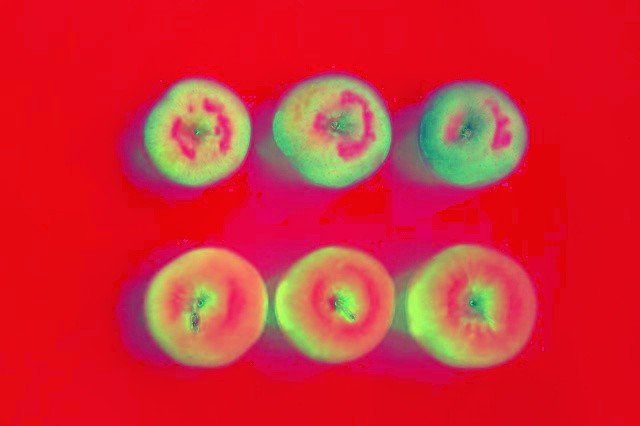
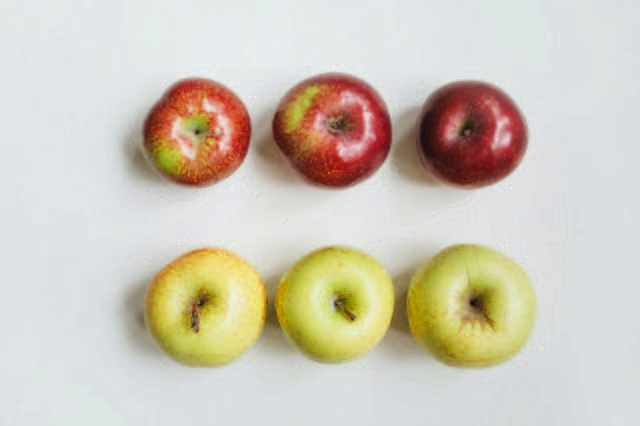