You can create an array in NumPy with the following code. If you want to learn Python then I will highly recommend you to read This Book.
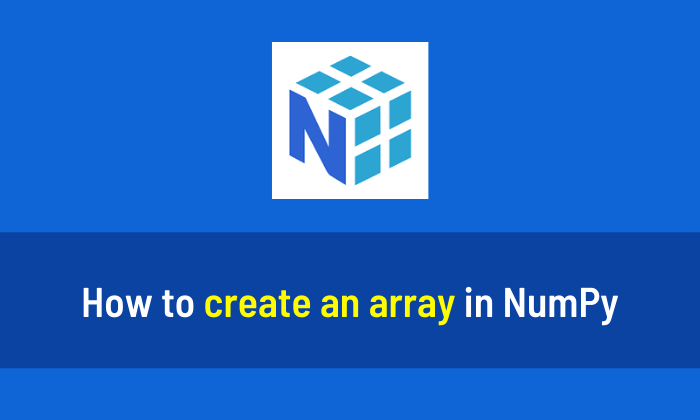
Example 1: 0-D Array
import numpy as np a = np.array(55) print(a)
55
Example 2: 1-D Array
import numpy as np a = np.array([1,2,3,4]) print(a)
[1 2 3 4]
Example 3: 2-D Array
import numpy as np a = np.array([[1, 2, 3, 4], [5, 6, 7, 8]]) print(a)
[[1 2 3 4] [5 6 7 8]]
Example 4: 3-D Array
import numpy as np a = np.array([[[1, 2, 3, 4], [5, 6, 7, 8]], [[1, 2, 3, 4], [5, 6, 7, 8]]]) print(a)
[[[1 2 3 4] [5 6 7 8]] [[1 2 3 4] [5 6 7 8]]]
Example 5: Higher Dimensional Array
import numpy as np a = np.array([1, 2, 3, 4], ndmin=6) print(a)
[[[[[[1 2 3 4]]]]]]
People are also reading:
What is Computer Vision? Examples, Applications, Techniques
Top 10 Computer Vision Books with Python
Books for Machine Learning (ML)