You can delete an element in the NumPy array with the following code. If you want to learn Python, I highly recommend reading This Book.
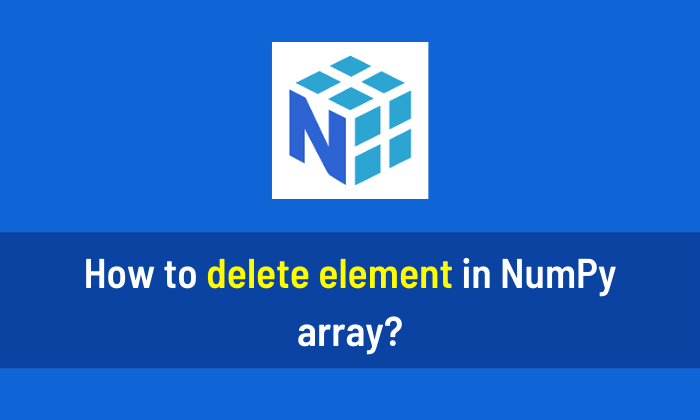
Example 1
import numpy as np a = np.array([5, 10, 15, 20, 25, 30, 35, 40]) index = [2, 4, 5] b = np.delete(a, index) print(b)
[ 5 10 20 35 40]
Example 2
import numpy as np a = np.array([5, 10, 15, 20, 25, 30, 35, 40]) b = np.array([15,25,30]) c = np.setdiff1d(a,b) print(c)
[ 5 10 20 35 40]
Example 3
a = np.array([5, 10, 15, 20, 25, 30, 35, 40]) b = np.delete(a, [2,4,5]) print(b)
[ 5 10 20 35 40]
Example 4
import numpy as np a = np.array([[1,2,3],[4,5,6]]) b = np.delete(a, [2,5]) print(b)
[1 2 4 5]
People are also reading:
What is Computer Vision? Examples, Applications, Techniques
Books for Machine Learning (ML)