You can concatenate two arrays in NumPy with the following code. If you want to learn Python, I highly recommend reading This Book.
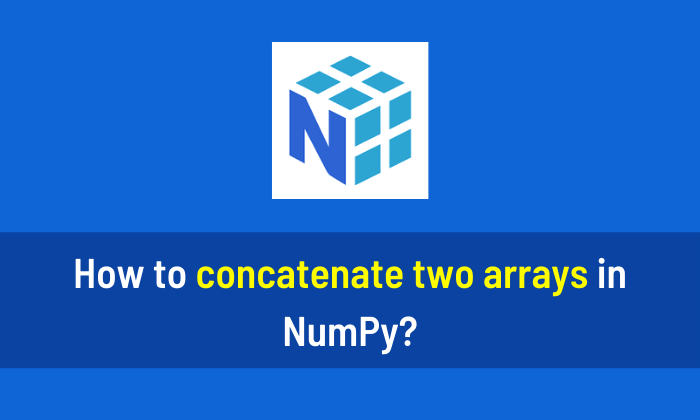
Example 1
In this example, I will concatenate two arrays row-wise.
# Import NumPy library import numpy as np # Make NumPy arrays a = np.array([[5, 10], [15, 20]]) b = np.array([[100, 200]]) # Concatenate arrays row wise print(np.concatenate((a, b), axis=0))
Output:
[[ 5 10] [ 15 20] [100 200]]
Example 2
In this example, I will concatenate two arrays column-wise.
# Import NumPy library import numpy as np # Make NumPy arrays a = np.array([[5, 10], [15, 20]]) b = np.array([[100, 200]]) # Concatenate arrays Column wise print(np.concatenate((a, b.T), axis=1))
Output:
[[ 5 10 100] [ 15 20 200]]
Example 3
# Import NumPy library import numpy as np # Make NumPy arrays a = np.array([[5, 10], [15, 20]]) b = np.array([[100, 200]]) # Concatenate arrays print(np.concatenate((a, b), axis=None))
Output:
[ 5 10 15 20 100 200]