You can use the append function in the NumPy array with the following code. If you want to learn Python, I highly recommend reading This Book.
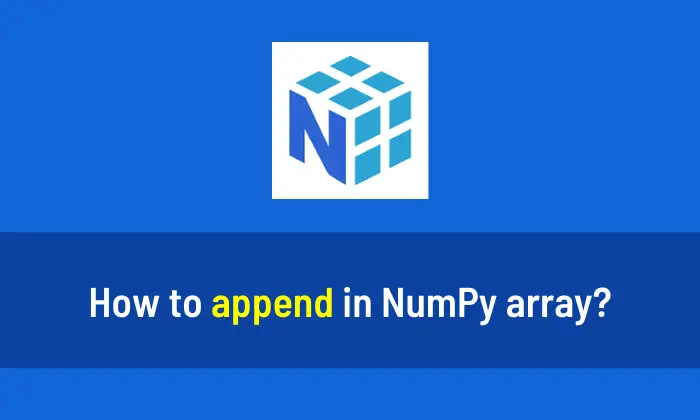
Example 1: How to append two NumPy arrays
import numpy as np a = np.array([1, 2, 3]) b = np.array([4, 5, 6]) c = np.append(a, b) print(c)
[1 2 3 4 5 6]
Example 2: How to append an element to the NumPy array
import numpy as np a = np.array([1, 2, 3]) b = np.append(a, 6) print(b)
[1 2 3 6]
Example 3: How to append column in NumPy array
import numpy as np a = np.array([[1, 2],[3, 4],[5,6]]) b = [[7],[8],[9]] c = np.append(a, b, axis=1) print(c)
[[1 2 7] [3 4 8] [5 6 9]]
Example 4: How to append row in NumPy array
import numpy as np a = np.array([[1, 2, 3],[4, 5, 6]]) b = [[7, 8, 9]] c = np.append(a, b, axis=0) print(c)
[[1 2 3] [4 5 6] [7 8 9]]
People are also reading:
What is Computer Vision? Examples, Applications, Techniques
Books for Machine Learning (ML)