You can calculate Euclidean distance in Python using NumPy with the following code. If you want to learn Python, I highly recommend reading This Book.
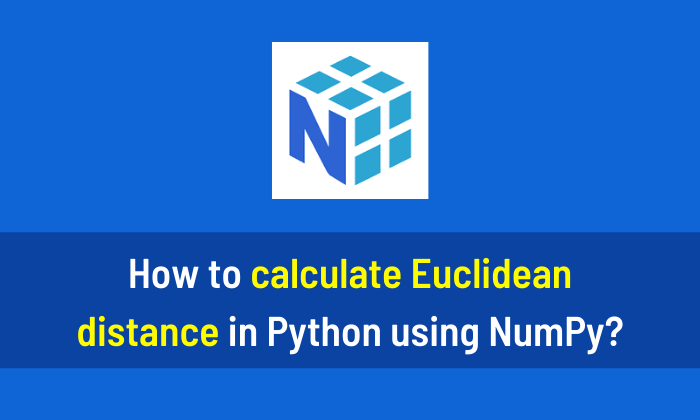
Method 1
import numpy as np A = np.array((3, 2)) B = np.array((4, 1)) Distance = np.linalg.norm(A - B) print(Distance)
1.4142135623730951
Method 2
import numpy as np A = np.array((3, 2)) B = np.array((4, 1)) C = A - B D = np.dot(C.T, C) Distance = np.sqrt(D) print(Distance)
1.4142135623730951
Method 3
import numpy as np A = np.array((3, 2)) B = np.array((4, 1)) C = np.sum(np.square(A - B)) Distance = np.sqrt(C) print(Distance)
1.4142135623730951
People are also reading:
What is Computer Vision? Examples, Applications, Techniques
Books for Machine Learning (ML)