You can add a row in the NumPy array with the following code. If you want to learn Python, I highly recommend reading This Book.
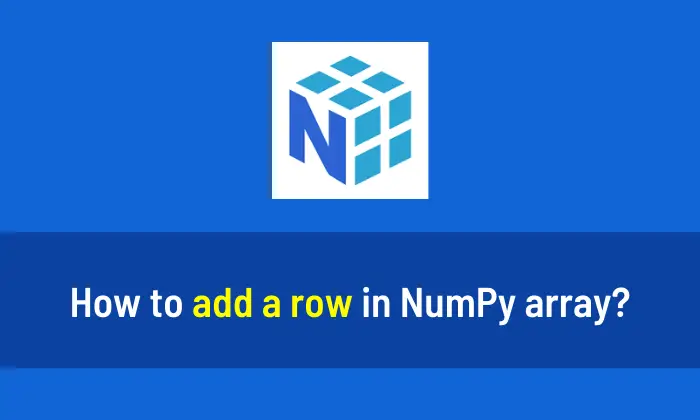
Method 1: Add a row using the insert method
import numpy as np # Original Numpy Array a = np.array([[1, 2, 3],[4, 5, 6],[7, 8, 9]]) # New Row b = [10, 20, 30] # Add new row using insert method c = np.insert(a, 2, b, axis=0) # Display new array print(c)
Output
[[ 1 2 3] [ 4 5 6] [10 20 30] [ 7 8 9]]
Method 2: Add a row using the append method
import numpy as np # Original Numpy Array a = np.array([[1, 2, 3],[4, 5, 6]]) # New Row b = [[10, 15, 20]] # Add new row using append method c = np.append(a, b, axis=0) # Display new array print(c)
Output
[[ 1 2 3] [ 4 5 6] [10 15 20]]
People are also reading:
What is Computer Vision? Examples, Applications, Techniques
Books for Machine Learning (ML)