You can check whether two NumPy arrays are equal with the following code. If you want to learn Python, I highly recommend reading This Book.
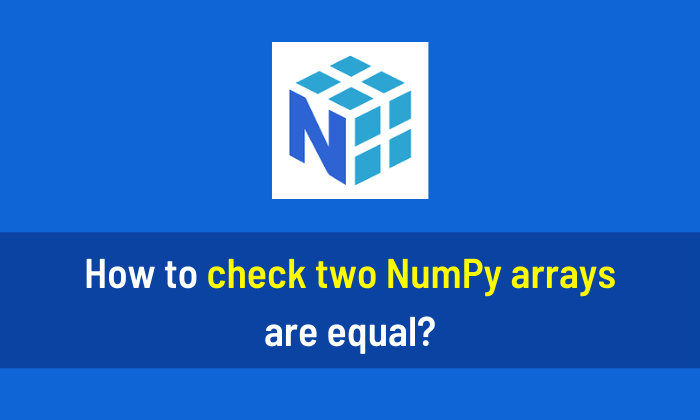
If NumPy is not installed, first install it using this code.
pip install numpy
Example 1
# Import the NumPy Library import numpy as np # Initialize the arrays a = np.array([[5, 10], [15, 20]]) b = np.array([[5, 10], [15, 20]]) # Check whether these arrays are equal print((a==b).all())
Output:
True
Example 2
# Import the NumPy Library import numpy as np # Initialize the arrays a = np.array([[5, 10], [15, 20]]) b = np.array([[2, 4], [6, 8]]) # Check whether these arrays are equal print((a==b).all())
Output:
False