You can reshape the NumPy array with the following code. If you want to learn Python, I highly recommend reading This Book.
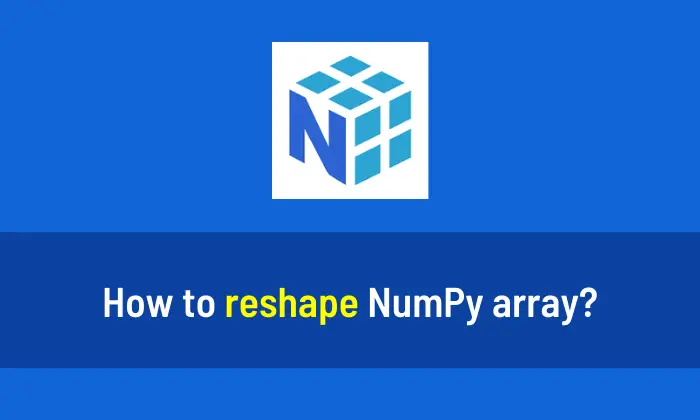
import numpy as np a = np.array([[1, 2, 3],[4, 5, 6]]) # Display array print(a) # Display shape of array print(a.shape) # Now reshape the above array into different possible shapes b = np.reshape(a,(3,2)) c = np.reshape(a,(1,6)) d = np.reshape(a,(6,1)) # Display new arrays print(b) print(c) print(d)
Output
[[1 2 3] [4 5 6]] (2, 3) [[1 2] [3 4] [5 6]] [[1 2 3 4 5 6]] [[1] [2] [3] [4] [5] [6]]