You can create random array in NumPy with the following code. If you want to learn Python then I will highly recommend you to read This Book.
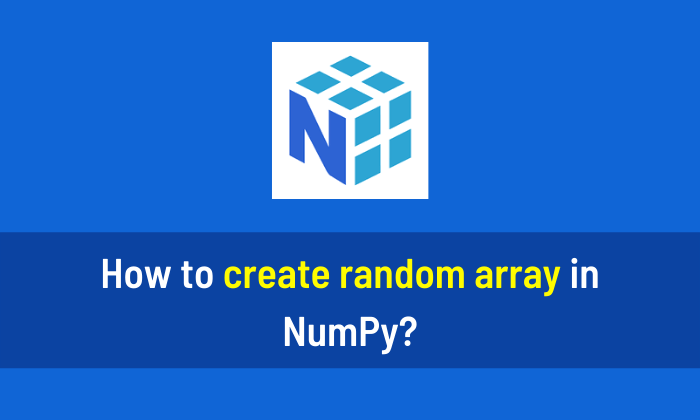
Example 1: Create 1d Random Array
It will create a 1d random array of 4 integers and the maximum integer limit is 50. The output will be different everytime because it is random generation.
from numpy import random a=random.randint(50, size=(4)) print(a)
[45 49 40 16]
Example 2: Create 2d Random Array
from numpy import random a = random.randint(50, size=(2, 4)) print(a)
[[43 1 48 1] [ 4 41 4 21]]
Example 3: (Float 1d Random Array)
It will create a 1d random array with 6 floating numbers. Every number will be in between 0 and 1.
from numpy import random a = random.rand(6) print(a)
[0.61470267 0.35447488 0.07999328 0.53609936 0.27370695 0.64716377]
Example 4: (Float 2d Random Array)
from numpy import random a = random.rand(4, 3) print(a)
[[9.49824158e-01 8.92868712e-01 2.81350709e-01] [9.41218138e-01 8.30483746e-01 5.67658690e-04] [4.70797102e-01 8.49414115e-01 3.78735775e-03] [1.46419094e-01 5.67291743e-01 9.74043337e-01]]
People are also reading:
What is Computer Vision? Examples, Applications, Techniques
Top 10 Computer Vision Books with Python
Books for Machine Learning (ML)