You can find NaN in the NumPy array with the following code. If you want to learn Python then I will highly recommend you to read This Book.
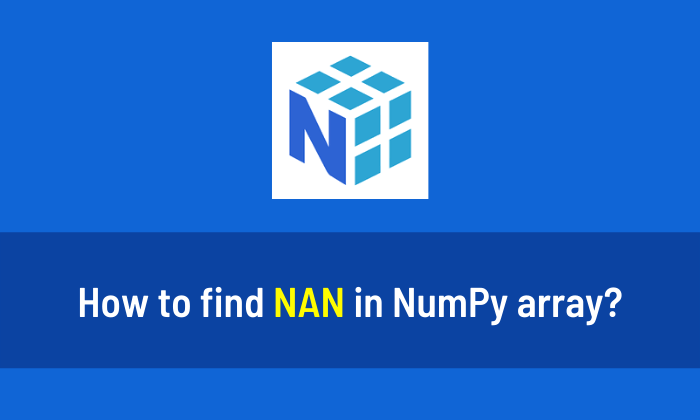
How to check is there any NaN in NumPy array?
import numpy as np a = np.array([1, 2, 3, np.nan, 5, np.nan]) print(np.isnan(a)) # Output is [False False False True False True]
How to find index of NaN in NumPy array?
import numpy as np a = np.array([1, 2, 3, np.nan, 5, np.nan]) print(np.argwhere(np.isnan(a))) # Output is [[3] [5]]
How to find total number of NaN values in NumPy array?
import numpy as np a = np.array([1, 2, 3, np.nan, 5, np.nan]) print(np.count_nonzero(np.isnan(a))) # Output is 2
How to find total number of Non NaN Values in NumPy array?
import numpy as np a = np.array([1, 2, 3, np.nan, 5, np.nan]) print(np.count_nonzero(~np.isnan(a))) # Output is 4
People are also reading:
What is Computer Vision? Examples, Applications, Techniques
Top 10 Computer Vision Books with Python
Books for Machine Learning (ML)