You can count values in the NumPy array with the following code. If you want to learn Python, I highly recommend reading This Book.
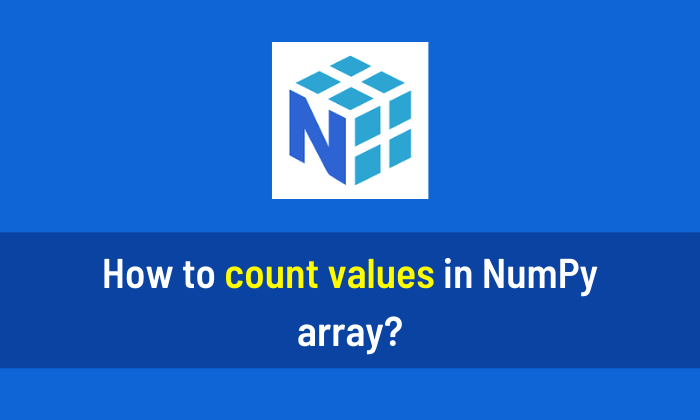
Method 1
import numpy as np a = np.array([5, 10, 15, 5, 5, 15, 5]) print(np.count_nonzero(a == 5)) print(np.count_nonzero(a == 10)) print(np.count_nonzero(a == 15))
4 1 2
Method 2
import numpy as np b = np.array([5, 10, 15, 5, 5, 15, 5]) (unique, counts) = np.unique(b, return_counts=True) print(unique, counts)
[ 5 10 15] [4 1 2]
Method 3
import collections, numpy a = numpy.array([5, 10, 15, 5, 5, 15, 5]) print(collections.Counter(a))
Counter({5: 4, 15: 2, 10: 1})
Method 4
import numpy as np a = np.array([5, 10, 15, 5, 5, 15, 5]) print((a == 5).sum()) print((a == 10).sum()) print((a == 15).sum())
4 1 2
Count all values of the NumPy array
import numpy as np a = np.array([5, 10, 15, 5, 5, 15, 5]) print(np.count_nonzero(a))
7
People are also reading:
What is Computer Vision? Examples, Applications, Techniques
Books for Machine Learning (ML)