You can count occurrences of elements in an array with the following code. If you want to learn Python, I highly recommend reading This Book.
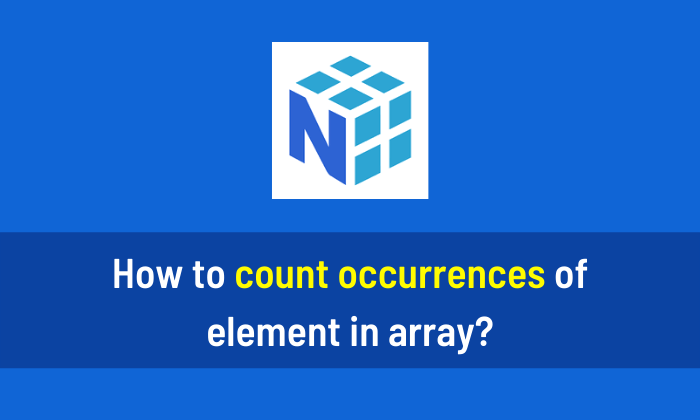
Method 1
import numpy as np a = np.array([1, 2, 3, 4, 1, 1, 2, 1, 4, 4, 1]) print(np.count_nonzero(a == 1)) print(np.count_nonzero(a == 2)) print(np.count_nonzero(a == 3)) print(np.count_nonzero(a == 4))
5 2 1 3
Method 2
import numpy as np b = np.array([1, 2, 3, 4, 1, 1, 2, 1, 4, 4, 1]) (unique, counts) = np.unique(b, return_counts=True) print(unique, counts)
[1 2 3 4] [5 2 1 3]
Method 3
import collections, numpy a = numpy.array([1, 2, 3, 4, 1, 1, 2, 1, 4, 4, 1]) print(collections.Counter(a))
Counter({1: 5, 4: 3, 2: 2, 3: 1})
Method 4
import numpy as np a = np.array([1, 2, 3, 4, 1, 1, 2, 1, 4, 4, 1]) print((a == 1).sum()) print((a == 2).sum()) print((a == 3).sum()) print((a == 4).sum())
5 2 1 3
People are also reading:
What is Computer Vision? Examples, Applications, Techniques
Books for Machine Learning (ML)