You can convert Pandas DataFrame to NumPy array with the following methods. If you want to learn Python, I highly recommend reading This Book.
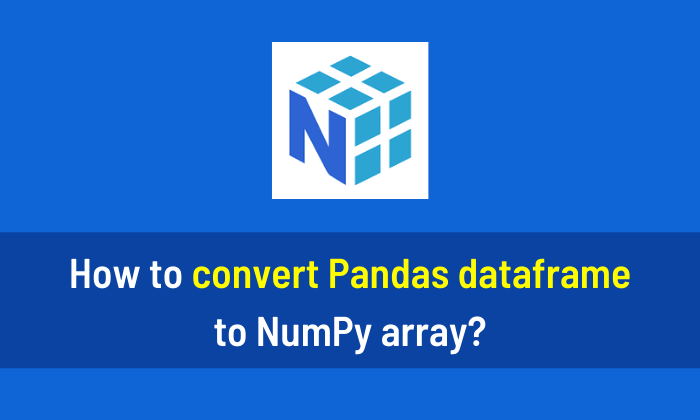
Method 1
In method 1, I will use the df.to_numpy() method for conversion.
# Import the required libraries import numpy as np import pandas as pd # Make a Pandas Dataframe df = pd.DataFrame(data={'column1': [5, 10, 15], 'column2': [25, 30, 35], 'column3': [70, 80, 90]}, index=['row1', 'row2', 'row3']) # Display Pandas Dataframe print(df) # Convert the entire DataFrame into NumPy array and display print(df.to_numpy()) # Convert specific columns into NumPy array and display print(df[['column1', 'column3']].to_numpy())
Output:
column1 column2 column3 row1 5 25 70 row2 10 30 80 row3 15 35 90 [[ 5 25 70] [10 30 80] [15 35 90]] [[ 5 70] [10 80] [15 90]]
Example 2
# Import the required libraries import numpy as np import pandas as pd # Read the data data = pd.read_csv("heart.csv") # Display type of data print(type(data)) # Convert the entire dataframe into NumPy array b = data.to_numpy() # Now Display type after conversion print(type(b))
Output:
<class 'pandas.core.frame.DataFrame'> <class 'numpy.ndarray'>
Method 2
In method 2, I will use the df.values method for conversion.
# Import the required libraries import numpy as np import pandas as pd # Make a Pandas Dataframe df = pd.DataFrame(data={'column1': [5, 10, 15], 'column2': [25, 30, 35], 'column3': [70, 80, 90]}, index=['row1', 'row2', 'row3']) # Display Pandas Dataframe print(df) print(df.values)
column1 column2 column3 row1 5 25 70 row2 10 30 80 row3 15 35 90 [[ 5 25 70] [10 30 80] [15 35 90]]
Example 2
# Import the required libraries import numpy as np import pandas as pd # Read the data data = pd.read_csv("heart.csv") # Display type of data print(type(data)) # Convert the entire dataframe into NumPy array b = df.values # Now Display type after conversion print(type(b))
Output:
<class 'pandas.core.frame.DataFrame'> <class 'numpy.ndarray'>
People are also reading:
What is Computer Vision? Examples, Applications, Techniques
Books for Machine Learning (ML)