You can concatenate arrays in the NumPy with the following code. If you want to learn Python, I highly recommend reading This Book.
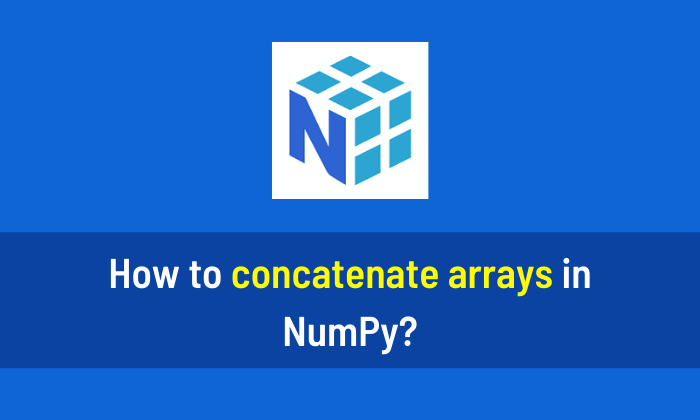
Example 1
import numpy as np a = np.array([[5, 10], [15, 20]]) b = np.array([[50, 100]]) print(np.concatenate((a, b), axis=0))
Output
[[ 5 10] [ 15 20] [ 50 100]]
Example 2
import numpy as np a = np.array([[5, 10], [15, 20]]) b = np.array([[50, 100]]) print(np.concatenate((a, b.T), axis=1))
Output
[[ 5 10 50] [ 15 20 100]]
Example 3
import numpy as np a = np.array([[5, 10], [15, 20]]) b = np.array([[50, 100]]) print(np.concatenate((a, b), axis=None))
Output
[ 5 10 15 20 50 100]
People are also reading:
What is Computer Vision? Examples, Applications, Techniques
Books for Machine Learning (ML)