You can append two arrays in the NumPy with the following code. If you want to learn Python, I highly recommend reading This Book.
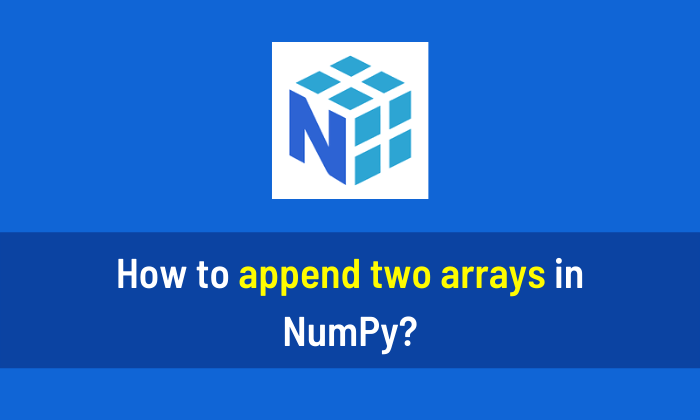
Example 1
import numpy as np a = np.array([5, 10, 15]) b = np.array([1, 2, 3]) c = np.append(a, b) print(c)
Output
[ 5 10 15 1 2 3]
Example 2
import numpy as np a = np.array([[5, 10, 15],[20, 25, 30]]) b = np.array([[1, 2, 3],[4, 5, 6]]) c = np.append(a, b) print(c)
[ 5 10 15 20 25 30 1 2 3 4 5 6]
Example 3
import numpy as np a = np.array([[5, 10, 15],[20, 25, 30]]) b = np.array([[1, 2, 3],[4, 5, 6]]) c = np.append(a, b,axis=0) print(c)
[[ 5 10 15] [20 25 30] [ 1 2 3] [ 4 5 6]]
Example 4
import numpy as np a = np.array([[5, 10, 15],[20, 25, 30]]) b = np.array([[1, 2, 3],[4, 5, 6]]) c = np.append(a, b,axis=1) print(c)
[[ 5 10 15 1 2 3] [20 25 30 4 5 6]]
People are also reading:
What is Computer Vision? Examples, Applications, Techniques
Books for Machine Learning (ML)