You can add a column in the NumPy array with the following code. If you want to learn Python, I highly recommend reading This Book.
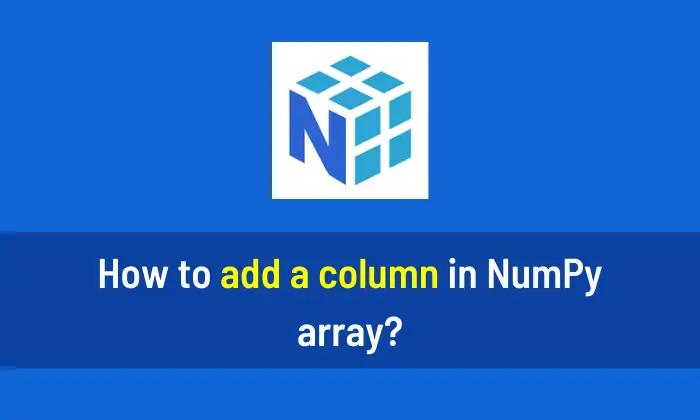
Method 1: Insert
import numpy as np # Original Numpy Array a = np.array([[1, 2, 3],[4, 5, 6],[7, 8, 9]]) # New Column b = [10, 20, 30] # Add new column using append method c = np.insert(a, 1, b, axis=1) # Display new array print(c)
Output
[[ 1 10 2 3] [ 4 20 5 6] [ 7 30 8 9]]
Method 2: Append
import numpy as np # Original Numpy Array a = np.array([[1, 2, 3],[4, 5, 6],[7, 8, 9]]) # New Column b = [[10], [20], [30]] # Add new column using append method c = np.append(a, b, axis=1) # Display new array print(c)
Output
[[ 1 2 3 10] [ 4 5 6 20] [ 7 8 9 30]]
People are also reading:
What is Computer Vision? Examples, Applications, Techniques
Books for Machine Learning (ML)