You can easily access columns in the NumPy array with the following code. If you want to learn Python, I highly recommend reading This Book.
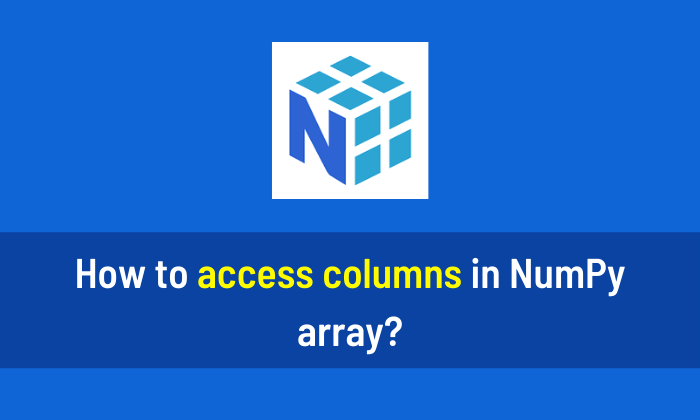
Method 1: Using Slices
In this method, I will use slices to access single, and multiple columns. The index starts from 0.
Example 1: Access single column
# Import NumPy library import numpy as np # Make a NumPy array a = np.array([[1, 2, 3, 4, 5], [2, 4, 6, 8, 10], [3, 4, 5, 6, 7]]) # Display 4th Column using Slices print(a[:,3])
[4 8 6]
Example 2: Access sequential multiple columns
# Import NumPy library import numpy as np # Make a NumPy array a = np.array([[1, 2, 3, 4, 5], [2, 4, 6, 8, 10], [3, 4, 5, 6, 7]]) # Display 4th and 5th Column using Slices print(a[:,3:5])
[[ 4 5] [ 8 10] [ 6 7]]
Example 3: Access random multiple columns
# Import NumPy library import numpy as np # Make a NumPy array a = np.array([[1, 2, 3, 4, 5], [2, 4, 6, 8, 10], [3, 4, 5, 6, 7]]) # Display 2nd and 5th Column using Slices print(a[:,[1,4]])
[[ 2 5] [ 4 10] [ 4 7]]
Method 2: Using Ellipsis
In this method, I will display the 5th column of the NumPy array using ellipsis. The index starts from 0.
# Import NumPy library import numpy as np # Make a NumPy array a = np.array([[1, 2, 3, 4, 5], [2, 4, 6, 8, 10], [3, 4, 5, 6, 7]]) # Display 5th Column using Ellipsis print(a[..., 4])
[ 5 10 7]
People are also reading:
What is Computer Vision? Examples, Applications, Techniques
Books for Machine Learning (ML)