You can easily plot a Pie Chart in Seaborn with the following code. The given examples with solutions will help you to understand how to plot a Pie Chart in Seaborn. I highly recommend the “Python Crash Course Book” to learn Python.
Example 1: Simple Pie Chart in Seaborn
# Import the required libraries import matplotlib.pyplot as plt import seaborn as sns # Data data = [25, 15, 25, 5, 30] # Labels labels = ['A', 'B', 'C', 'D', 'E'] # Create Pie Chart plt.pie(data, labels = labels, autopct='%.0f%%') # Display Pie Chart plt.show()
Output:
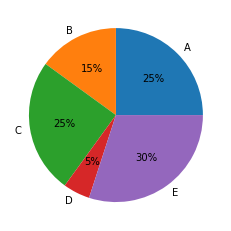
Example 2: Change Colors of Pie Chart
# Import the required libraries import matplotlib.pyplot as plt import seaborn as sns # Data data = [25, 15, 25, 5, 30] # Labels labels = ['A', 'B', 'C', 'D', 'E'] # Change Color sns.set_palette(sns.color_palette("rocket")) # Create Pie Chart plt.pie(data, labels = labels, autopct='%.0f%%') # Display Pie Chart plt.show()
Output:
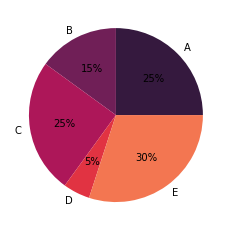
Example 3: Define Own Colors and Explode Parameter
# Import the required libraries import matplotlib.pyplot as plt import seaborn as sns # Define color codes colors = ["#FFA500","#00FF00","#FFFF00","#FFCC99","#0000FF"] # Data data = [25, 15, 25, 5, 30] # Labels labels = ['A', 'B', 'C', 'D', 'E'] # Mention Explode Values explode = [0, 0, 0, 0, 0.1] # Set Color Palette sns.set_palette(sns.color_palette(colors)) # Create Pie Chart plt.pie(data, labels = labels, autopct='%.0f%%', explode=explode) # Display Chart plt.show()
Output:
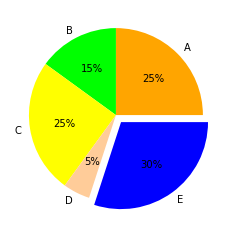