You can implement linear regression using the sklearn library by following the given steps. If you want to learn Machine Learning then I will highly recommend you to read This Book.
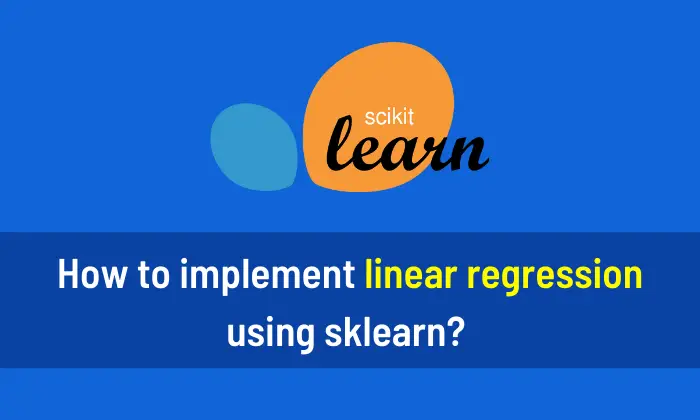
Step 1: Read the Data
Read the data from the location using Pandas library. In my case, “C:\Users\engrh\Desktop\ML” is the location and “house_price.csv” is the name of the file. Change it according to your data file and location.
import pandas as pd df = pd.read_csv('C:\\Users\\engrh\\Desktop\\ML\\house_price.csv')
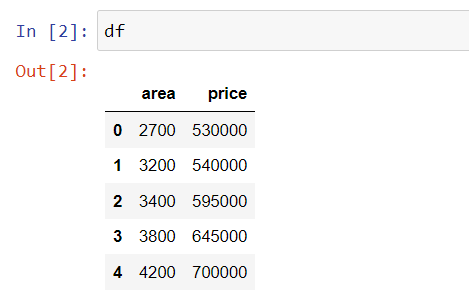
Step 2: Scatter Plot
Now draw the scatter plot to see the trend.
from matplotlib import pyplot as plt plt.title('Price of House at Given Area',fontsize =15) plt.xlabel('Area (sq.feet)',fontsize =15) plt.ylabel('Actual Given Price ($)',fontsize =15) plt.scatter(df.area,df.price,color='red',marker='*') plt.show()
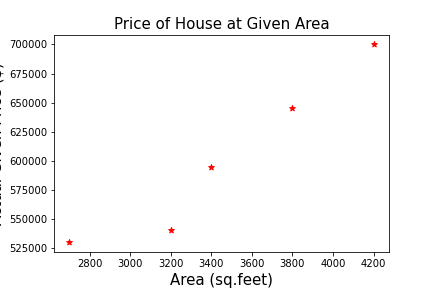
Step 3: Linear Regression using sklearn
Now implement Linear Regression using the sklearn library. First import the linear model from the sklearn then select the linear regression and store it in the variable named ‘model’. Now fit the linear regression on the data. The ‘area’ is the feature and the ‘price’ is our target variable.
from sklearn import linear_model model = linear_model.LinearRegression() model.fit(df[['area']],df['price'])
Step 4: Prediction
Now draw a line that our model predicted for given values.
plt.scatter(df.area,df.price,color='red',marker='*') plt.xlabel('Area(sq.feet)',fontsize =15) plt.ylabel('Price($)',fontsize =15) plt.plot(df.area,model.predict(df[['area']]),color='green') plt.show()
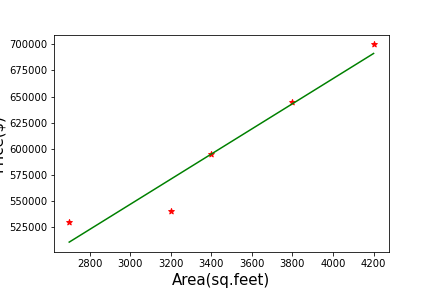
Now predict for a single value.
model.predict([[3300]]) #Output #array([582682.92682927])
Prediction on multiple values.
import numpy as np new_data = np.array([4500,5000,5500,6000,6500,7000]) new_data = new_data.reshape(6,1) model.predict(new_data)
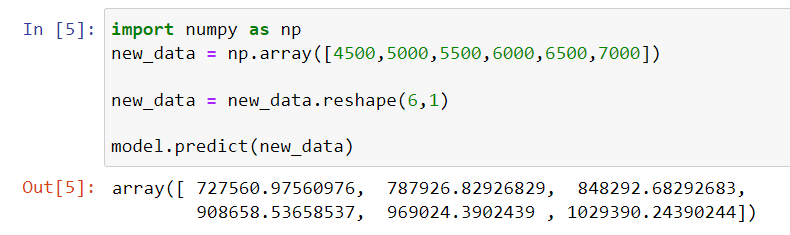
People are also reading:
Books for Machine Learning (ML)
What is Computer Vision? Examples, Applications, Techniques
Top 10 Computer Vision Books with Python